matlab 拉伸figure保持轴的坐标不变
时间: 2024-10-17 10:14:26 浏览: 33
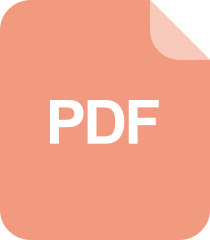
Matlab Figure 图形保存以及 Simulink 中 Scope 窗口坐标标注(label)的设置.pdf
在MATLAB中,当你对`figure`窗口进行缩放(如通过鼠标滚轮操作拉伸)时,通常轴的刻度会自动调整以适应新的窗口大小。如果你想要在拉伸figure的同时保持轴的坐标比例不变,可以使用`datetick`、`xlim`、`ylim`函数或者设置`units`属性来控制。
首先,你可以锁定轴的范围(xlim和ylim),这样无论窗口放大还是缩小,轴的边界值不会改变:
```matlab
% 定义固定轴限
xlim([start_x end_x])
ylim([start_y end_y])
% 或者,如果你有时间数据,可以使用datetick来保持日期刻度
xtick = datenum('your_date_range');
xlim(xtick(1):xtick(end));
```
其次,你可以设置`Units`属性为特定单位(如'inches'),这样缩放只会改变图像尺寸,而不是轴的比例:
```matlab
set(gca, 'Units', 'Inches'); % gca代表当前活动图
```
如果需要在每个窗口都应用这个设置,可以在创建`figure`时添加这个属性:
```matlab
fig = figure('Units', 'Inches');
```
阅读全文
相关推荐
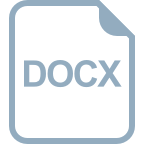
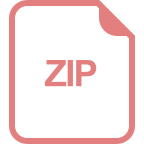
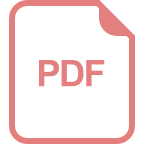
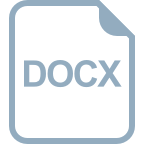
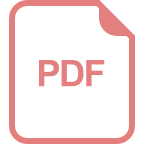
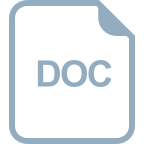
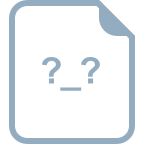
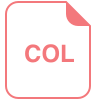
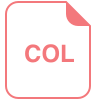
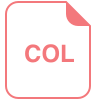
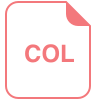





