reverse_string
时间: 2023-08-05 10:08:15 浏览: 118
Sure! To reverse a string, you can simply iterate through the characters of the string in reverse order and append them to a new string. Here's an example in Python:
```python
def reverse_string(string):
reversed_string = ""
for i in range(len(string) - 1, -1, -1):
reversed_string += string[i]
return reversed_string
# Example usage
string = "Hello, World!"
reversed_string = reverse_string(string)
print(reversed_string)
```
The output will be "!dlroW ,olleH".
相关问题
reverse_string(string+1)
"reverse_string(string+1)" 这样的表述似乎是一个函数调用,但是语法上有点问题,因为 "+" 操作符通常用于连接字符串而不是访问字符序列。如果这里的 "string+1" 是指从第二个字符开始遍历字符串,那么它更像是一个伪代码表示,真正的函数名应该是 "reverse_string" 或者 "reverse",接收一个字符串作为参数,然后返回反转后的字符串。
在许多编程语言中,如 Python 中,可以这样实现:
```python
def reverse_string(s):
return s[::-1]
```
这里 `s[::-1]` 表示从字符串末尾开始到开头取字符,步长为 -1,实现了字符串反转。
如果你是指 C++ 或 Java 这样的需要显式迭代的编程语言,可能会这样做:
```cpp
#include <iostream>
#include <string>
std::string reverse_string(const std::string& s) {
std::string reversed;
for (int i = s.length() - 1; i >= 0; i--) {
reversed += s[i];
}
return reversed;
}
// 或者 Java:
public String reverseString(String s) {
StringBuilder sb = new StringBuilder();
for (int i = s.length() - 1; i >= 0; i--) {
sb.append(s.charAt(i));
}
return sb.toString();
}
```
Write a Python function named "reverse_string" that takes a string as input and returns the reverse of the input string.
Sure! Here's a Python function named "reverse_string" that takes a string as input and returns the reverse of the input string:
```python
def reverse_string(input_str):
return input_str[::-1]
```
Here, we're using Python's string slicing notation to reverse the input string. The `[::-1]` slice means to take the entire string, but step backwards by 1 character at a time. This effectively reverses the order of the string.
阅读全文
相关推荐
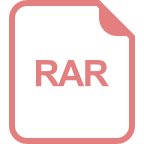












