C++如何使用RabbitMQ
时间: 2024-06-16 14:08:10 浏览: 176
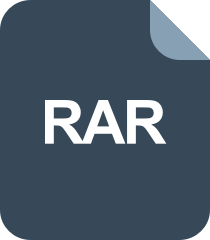
C++/QT 使用RabbitMQ
C++可以使用RabbitMQ的C++客户端库来与RabbitMQ进行交互。下面是使用RabbitMQ的C++客户端库的一些步骤和示例代码:
1. 首先,你需要在你的C++项目中导入RabbitMQ的C++客户端库。你可以通过在项目配置文件中添加相关的库和头文件路径来实现。例如,你可以在项目的.pro文件中添加以下内容[^2]:
```shell
TEMPLATE = app
CONFIG += console c++11
CONFIG -= app_bundle
CONFIG -= qt
SOURCES += \
main.cpp \
rabbitmqclient.cpp
HEADERS += \
rabbitmqclient.h
unix:!macx: LIBS += -L$$PWD/../../rabbitmq-c-0.9.0/build/librabbitmq/ -lrabbitmq
INCLUDEPATH += $$PWD/../../rabbitmq-c-0.9.0/build/librabbitmq
DEPENDPATH += $$PWD/../../rabbitmq-c-0.9.0/build/librabbitmq
unix:!macx: PRE_TARGETDEPS += $$PWD/../../rabbitmq-c-0.9.0/build/librabbitmq/librabbitmq.a
```
2. 在你的C++代码中,你可以使用RabbitMQ的C++客户端库提供的函数来连接RabbitMQ服务器、声明交换机、声明队列、绑定队列、发布消息和消费消息等操作。以下是一个简单的示例代码[^1]:
```cpp
#include <iostream>
#include <string>
#include <amqp.h>
int main() {
// 连接RabbitMQ服务器
amqp_connection_state_t conn = amqp_new_connection();
amqp_socket_t *socket = amqp_tcp_socket_new(conn);
amqp_socket_open(socket, "localhost", 5672);
// 创建一个通道
amqp_channel_open(conn, 1);
amqp_channel_confirm_select(conn, 1);
// 声明交换机
amqp_exchange_declare(conn, 1, amqp_cstring_bytes("my_exchange"), amqp_cstring_bytes("direct"), 0, 0, 0, 0, amqp_empty_table);
// 声明队列
amqp_queue_declare(conn, 1, amqp_cstring_bytes("my_queue"), 0, 0, 0, 0, amqp_empty_table);
// 绑定队列到交换机
amqp_queue_bind(conn, 1, amqp_cstring_bytes("my_queue"), amqp_cstring_bytes("my_exchange"), amqp_cstring_bytes("my_routing_key"), amqp_empty_table);
// 发布消息
std::string message = "Hello, RabbitMQ!";
amqp_basic_publish(conn, 1, amqp_cstring_bytes("my_exchange"), amqp_cstring_bytes("my_routing_key"), 0, 0, nullptr, amqp_cstring_bytes(message.c_str()));
// 消费消息
amqp_basic_consume(conn, 1, amqp_cstring_bytes("my_queue"), amqp_empty_bytes, 0, 1, 0, amqp_empty_table);
amqp_frame_t frame;
amqp_basic_deliver_t *deliver;
amqp_basic_properties_t *properties;
amqp_bytes_t body;
while (1) {
amqp_simple_wait_frame(conn, &frame);
if (frame.frame_type != AMQP_FRAME_METHOD) {
continue;
}
if (frame.payload.method.id != AMQP_BASIC_DELIVER_METHOD) {
continue;
}
deliver = (amqp_basic_deliver_t *)frame.payload.method.decoded;
amqp_simple_wait_frame(conn, &frame);
if (frame.frame_type != AMQP_FRAME_HEADER) {
continue;
}
properties = (amqp_basic_properties_t *)frame.payload.properties.decoded;
body = amqp_bytes_malloc(properties->body_size);
amqp_simple_wait_frame(conn, &frame);
if (frame.frame_type != AMQP_FRAME_BODY) {
continue;
}
memcpy(body.bytes, frame.payload.body_fragment.bytes, frame.payload.body_fragment.len);
std::string message((char *)body.bytes, body.len);
std::cout << "Received message: " << message << std::endl;
amqp_bytes_free(body);
}
// 关闭连接
amqp_channel_close(conn, 1, AMQP_REPLY_SUCCESS);
amqp_connection_close(conn, AMQP_REPLY_SUCCESS);
amqp_destroy_connection(conn);
return 0;
}
```
请注意,以上示例代码仅为演示目的,实际使用时可能需要根据你的具体需求进行适当的修改。
阅读全文
相关推荐



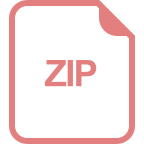
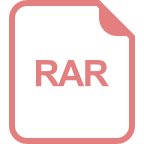
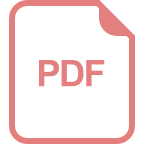
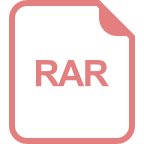
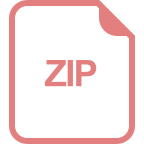
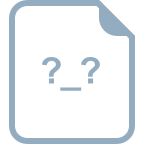
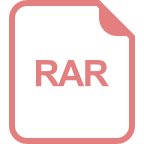
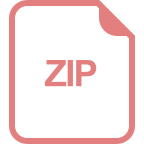
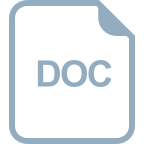




