写一个图书管理系统的类设计C++
时间: 2024-09-14 08:09:03 浏览: 13
在C++中,我们可以创建一个简单的图书管理系统,它包括基本的数据结构和几个类来表示图书馆、图书以及相关的操作。这里是一个基础的设计示例:
```cpp
// 定义一个图书类Book
class Book {
public:
// 构造函数
Book(string title, string author, int year) : title(title), author(author), publication_year(year) {}
// 属性
string getTitle() const { return title; }
string getAuthor() const { return author; }
int getPublicationYear() const { return publication_year; }
private:
string title;
string author;
int publication_year;
};
// 定义一个库存类Inventory
class Inventory {
public:
void addBook(Book book);
void removeBook(const string& title);
void displayBooks();
private:
map<string, Book> books; // 使用map存储书籍标题和对应的图书实例
};
// 实现Inventory类的方法
void Inventory::addBook(Book book) {
books[book.getTitle()] = book;
}
void Inventory::removeBook(const string& title) {
books.erase(title);
}
void Inventory::displayBooks() {
for (const auto& pair : books) {
cout << "Title: " << pair.first << ", Author: " << pair.second.getAuthor()
<< ", Publication Year: " << pair.second.getPublicationYear() << endl;
}
}
// 主程序入口
int main() {
Inventory library;
library.addBook(Book("The Catcher in the Rye", "J.D. Salinger", 1951));
library.addBook(Book("To Kill a Mockingbird", "Harper Lee", 1960));
// ... 进行其他操作
return 0;
}
```
这个设计中,`Book`类代表每本书的信息,而`Inventory`类负责管理图书的添加、移除和显示。通过这种方式,我们可以方便地扩展或修改图书管理系统,比如添加借阅功能或者用户信息。
相关推荐
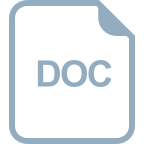
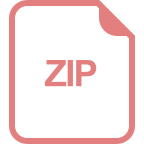
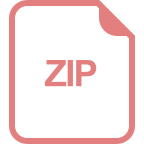














