C# 写一个方法,递归查找DATATABLE DtBom 找到对应的母子件关系,DtBom 有三个字段 母件编码,子件编码,子件用量,找到对应木子关系后转换成物料表,字段如下:BOM层级,物料编码,用量
时间: 2024-10-18 11:16:35 浏览: 18
在C#中,你可以编写一个递归函数来遍历`DataTable`(类似于数据库表格)并查找母子件关系。首先,假设你有一个名为`FindChildItems`的方法,它接受`DataTable DtBom`作为输入,以及两个参数`parentCode`(当前处理的母件编码)和`childItems`(用于存储子件信息的列表)。下面是示例代码:
```csharp
using System;
using System.Collections.Generic;
using System.Data;
public class BOMHelper
{
public static List<(int Level, string MaterialCode, int Usage)> FindChildItems(DataTable DtBom, string parentCode, List<(int, string, int)> childItems)
{
foreach (DataRow row in DtBom.Rows)
{
if (row.Field<string>("ParentCode") == parentCode)
{
string childCode = row.Field<string>("ChildCode");
int usage = Convert.ToInt32(row.Field<string>("SubItemUsage"));
// 如果已经有相同的子件,增加层级;否则新建项添加层级和用量
var existingChild = childItems.Find(c => c.Item2 == childCode);
if (existingChild != null)
{
existingChild.Item1++;
}
else
{
childItems.Add((1, childCode, usage));
}
// 递归查找子件的子件
childItems.AddRange(FindChildItems(DtBom, childCode, childItems));
}
}
return childItems;
}
}
// 使用示例
DataTable DtBom; // 假设已经填充数据的DataTable
List<(int, string, int)> bomData = BOMHelper.FindChildItems(DtBom, "", new List<(int, string, int)>());
```
这个方法会返回一个包含`(BOM层级, 物料编码, 用量)`三元组的列表。注意,这里假设了`SubItemUsage`字段可以转换为整数。
阅读全文
相关推荐
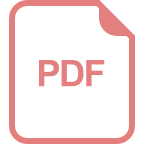
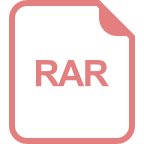
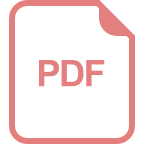
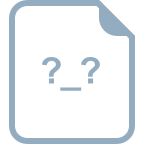
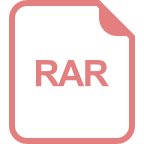
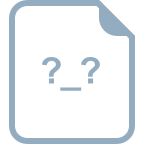
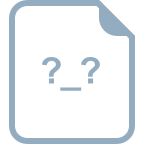
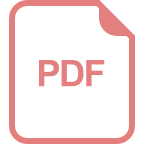
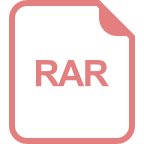
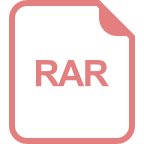
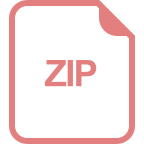
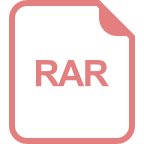
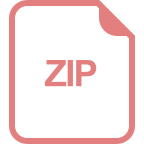
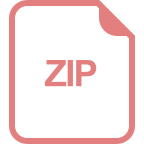
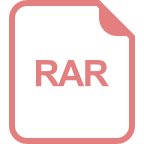
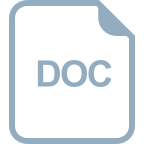