帮我写一个在第i个位置插入元素e的单链表的C语言程序
时间: 2024-09-18 20:10:53 浏览: 42
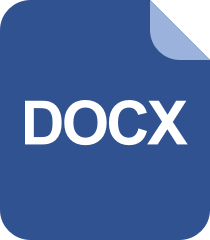
C语言程序设计课程设计题目.docx
在C语言中,你可以使用一个结构体表示链表节点,并编写函数来在指定位置插入新元素。首先,我们需要定义一个链表节点结构:
```c
#include <stdio.h>
#include <stdlib.h>
// 链表节点结构
typedef struct Node {
int data;
struct Node* next;
} Node;
// 在链表第i个位置插入元素e的函数
void insertNode(Node** head, int i, int e) {
// 如果链表为空或索引越界
if (*head == NULL || i <= 0) {
printf("Invalid insertion position.\n");
return;
}
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = e; // 新节点的数据
if (i == 1) { // 插入在第一个位置
newNode->next = *head;
*head = newNode;
} else {
Node* current = *head;
for (int j = 1; j < i - 1 && current != NULL; j++) {
current = current->next;
}
if (current != NULL) { // 找到插入位置
newNode->next = current->next;
current->next = newNode;
} else {
printf("Index out of range.\n");
}
}
}
// 主函数示例
int main() {
Node* head = NULL; // 初始化链表头指针
insertNode(&head, 2, 5); // 在第二个位置插入元素5
// ... 其他操作...
return 0;
}
```
这个程序会在第`i`个位置插入新的整数`e`。注意,链表的索引从1开始计数。如果链表为空或者插入的位置不合理(例如大于链表长度),函数会给出错误提示。
阅读全文
相关推荐
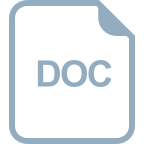
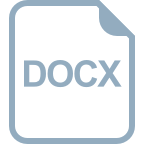
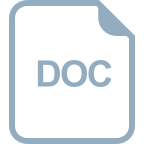
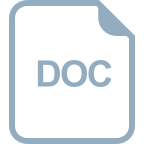
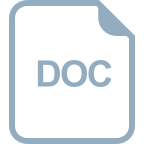
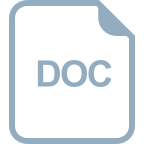
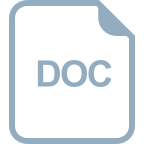
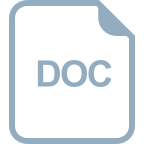
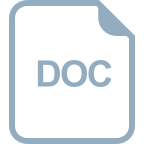






