string.erase
时间: 2023-10-03 22:07:38 浏览: 37
`string.erase`是用于删除字符串中的字符或一段字符的函数。它可以接受一个参数,指定要删除的字符的位置或范围。
如果只提供一个参数,那么它将从指定位置开始删除到字符串的末尾。如果提供两个参数,第一个参数是要删除的起始位置,第二个参数是要删除的字符的数量。
以下是一个示例:
```cpp
#include <iostream>
#include <string>
int main() {
std::string str = "Hello, World!";
str.erase(7); // 从位置7开始删除字符
std::cout << str << std::endl; // 输出: "Hello, "
std::string str2 = "Hello, World!";
str2.erase(7, 6); // 从位置7开始删除6个字符
std::cout << str2 << std::endl; // 输出: "Hello!"
return 0;
}
```
在上面的示例中,`str.erase(7)`删除了字符串 `str` 中从位置7开始的所有字符,结果为 `"Hello, "`。而`str2.erase(7, 6)`删除了字符串 `str2` 中从位置7开始的6个字符,结果为 `"Hello!"`。
相关问题
c++ string.erase
The `string.erase()` function is a member function of the C++ standard library's `string` class. It is used to remove a portion of the string, starting at a specified position and for a specified length.
Syntax:
```
string.erase(position, length);
```
Here, `position` is an integer value that specifies the starting position from where the characters are to be removed. `length` is also an integer value that specifies the number of characters to be removed.
Example:
```c++
#include <iostream>
#include <string>
int main()
{
std::string str = "Hello, World!";
// remove the first 6 characters
str.erase(0, 6);
std::cout << str << std::endl; // output: World!
return 0;
}
```
In the above example, the `erase()` function is used to remove the first 6 characters from the string `str`. The resulting string is then output to the console.
string.erase()
`string.erase()` 是一个 C++ 中的字符串操作函数,用于删除字符串中的一部分或全部字符。该函数有多种重载形式,可以接受不同的参数,以实现不同的删除操作。
以下是 `string.erase()` 函数最常用的重载形式:
```c++
string& erase(size_t pos = 0, size_t len = npos);
```
该函数从 `pos` 位置开始删除 `len` 个字符。如果 `len` 参数被省略或设置为 `npos`(`string::npos` 是一个常量,表示无穷大),则删除从 `pos` 到字符串末尾的所有字符。
例如,以下代码将删除字符串 `str` 中从位置 2 开始的 3 个字符:
```c++
std::string str = "Hello, world!";
str.erase(2, 3); // 删除从位置 2 开始的 3 个字符
```
最终结果为:`He, world!`。
相关推荐
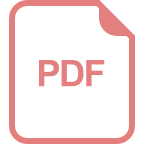
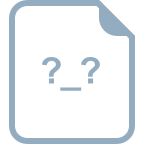
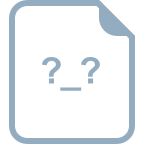












