快速排序随机算法代码
时间: 2024-07-31 13:01:13 浏览: 45
快速排序是一种常用的排序算法,其基本思想是通过一趟排序将待排记录分隔成独立的两部分,其中一部分记录的关键字均比另一部分的关键字小,然后分别对这两部分记录继续进行排序,直到整个序列有序。这里是一个基于随机化选择枢轴元素的快速排序Python代码示例:
```python
import random
def quicksort(arr):
if len(arr) <= 1:
return arr
# 随机选择枢轴
pivot_index = random.randint(0, len(arr) - 1)
pivot = arr[pivot_index]
# 将数组分为三部分:小于、等于、大于枢轴的部分
less = [x for x in arr[:pivot_index] if x < pivot]
equal = [x for x in arr[pivot_index:] if x == pivot]
greater = [x for x in arr[pivot_index + 1:] if x > pivot]
# 递归地排序小部分和大部分
return quicksort(less) + equal + quicksort(greater)
# 测试代码
arr = [3,6,8,10,1,2,1]
sorted_arr = quicksort(arr)
print("Sorted array:", sorted_arr)
相关问题
机器人快速排序随机算法代码
机器人快速排序是一种基于快速排序算法的变体,通常在处理不确定输入顺序的情况时采用,通过引入随机化元素来提高效率。以下是简单的Python代码示例,展示了如何实现机器人快速排序:
```python
import random
def quicksort_randomized(arr):
if len(arr) <= 1:
return arr
pivot_index = random.randint(0, len(arr) - 1)
pivot = arr[pivot_index]
arr, arr[pivot_index] = arr[pivot_index], arr # 将基准元素放在第一个位置
smaller, equal, larger = [], [], []
for element in arr[1:]:
if element < pivot:
smaller.append(element)
elif element == pivot:
equal.append(element)
else:
larger.append(element)
return quicksort_randomized(smaller) + equal + quicksort_randomized(larger)
# 示例用法
arr = [9, 5, 1, 7, 3, 6, 8, 4, 2]
sorted_arr = quicksort_randomized(arr)
print("Sorted array:", sorted_arr)
快速排序随机化算法c++
快速排序随机化算法是一种改进的快速排序算法,它通过随机选择基准元素来避免最坏情况的发生,从而提高了算法的平均性能。下面是快速排序随机化算法的C++代码实现:
```C++
#include <iostream>
#include <cstdlib>
#include <ctime>
using namespace std;
void Init_Random(int arr[], int n) {
srand(time(NULL));
for (int i = 0; i < n; i++) {
arr[i] = rand() % 100;
}
}
void Show_Array(int arr[], int n) {
for (int i = 0; i < n; i++) {
cout << arr[i] << " ";
}
cout << endl;
}
int Partition(int arr[], int left, int right) {
int pivot = arr[left];
while (left < right) {
while (left < right && arr[right] >= pivot) {
right--;
}
arr[left] = arr[right];
while (left < right && arr[left] <= pivot) {
left++;
}
arr[right] = arr[left];
}
arr[left] = pivot;
return left;
}
void Quick_Sort(int arr[], int left, int right) {
if (left < right) {
int pivot_pos = Partition(arr, left, right);
Quick_Sort(arr, left, pivot_pos - 1);
Quick_Sort(arr, pivot_pos + 1, right);
}
}
int main() {
const int n = 10;
int arr[n];
Init_Random(arr, n);
cout << "Original array:" << endl;
Show_Array(arr, n);
Quick_Sort(arr, 0, n - 1);
cout << "Sorted array:" << endl;
Show_Array(arr, n);
return 0;
}
```
阅读全文
相关推荐
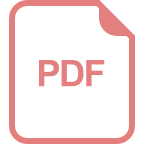
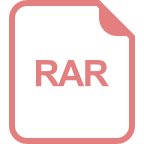
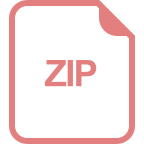













