kafka判断可以是否存在代码
时间: 2024-10-27 18:07:23 浏览: 20
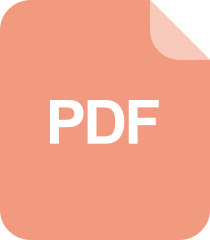
java面试笔记整理,包含java,redis,kafka等
在Apache Kafka中,判断一个特定的消息是否存在于某个主题(Topic)并不直接提供一个简单的API来实现。但你可以通过以下步骤间接实现:
1. **消费者读取**:创建一个消费者,订阅目标主题。然后,你可以不断地使用`poll()`方法尝试从主题中拉取消息,如果能得到该消息,说明存在。
```java
Properties props = ...;
KafkaConsumer<String, String> consumer = new KafkaConsumer<>(props);
consumer.subscribe(Arrays.asList(topicName));
while (true) {
ConsumerRecords<String, String> records = consumer.poll(Duration.ofMillis(100));
for (ConsumerRecord<String, String> record : records) {
if (record.key().equals(yourKey)) {
System.out.println("Message exists with key: " + record.key());
break; // 找到消息后退出循环
}
}
}
```
这里假设`yourKey`是你想要查找的关键字。
2. **元数据查询**:如果你关心的是主题的最新偏移量(offset)是否超过某个值,你可以查询元数据服务(Metadata Service)。但这个过程比较复杂,需要处理网络请求,并且不是实时的。
```java
AdminClient adminClient = AdminClient.create(props);
ListTopicsResult listTopicsResult = adminClient.listTopics();
List<Topic> topics = listTopicsResult.names();
for (Topic topic : topics) {
TopicDescription topicDesc = adminClient.describeTopics(Collections.singletonList(topic)).all().get(topic);
long latestOffset = topicDesc.partitions().values().stream()
.mapToInt(p -> p.leaderEpoch() == -1 ? p.highWatermark() : p.position())
.max().orElse(-1); // 如果有leader,则使用position;否则使用highWatermark
if (latestOffset > yourThreshold) {
System.out.println("Messages exist beyond the threshold.");
}
}
```
请注意,上述代码示例需要处理异常和适当的错误处理。另外,Kafka本身的设计不适合频繁地进行这类“是否存在”检查,因为它设计用于高效地推送大量消息,而非查询单个消息的状态。
阅读全文
相关推荐
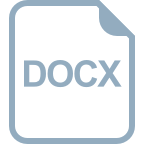
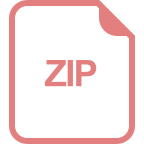


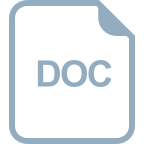
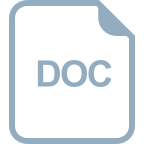
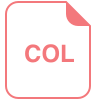
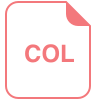
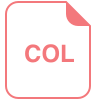
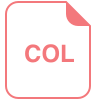
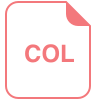
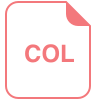
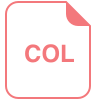
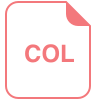
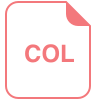


