c代码 spinlock
时间: 2024-03-23 21:33:44 浏览: 21
Spinlock是一种用于同步多线程访问共享资源的机制,它可以确保在任意时刻只有一个线程可以访问被保护的资源。在C语言中,可以使用自旋锁(spinlock)来实现这种机制。
自旋锁是一种忙等待的锁,当一个线程尝试获取自旋锁时,如果锁已经被其他线程占用,该线程会一直循环等待,直到锁被释放。自旋锁适用于保护临界区较小且被占用时间较短的情况。
以下是一个简单的C代码示例,展示了如何使用自旋锁:
```c
#include <stdio.h>
#include <pthread.h>
pthread_spinlock_t spinlock;
int shared_resource = 0;
void* thread_func(void* arg) {
pthread_spin_lock(&spinlock);
shared_resource++;
printf("Thread %ld: shared_resource = %d\n", (long)arg, shared_resource);
pthread_spin_unlock(&spinlock);
return NULL;
}
int main() {
pthread_t thread1, thread2;
pthread_spin_init(&spinlock, 0);
pthread_create(&thread1, NULL, thread_func, (void*)1);
pthread_create(&thread2, NULL, thread_func, (void*)2);
pthread_join(thread1, NULL);
pthread_join(thread2, NULL);
pthread_spin_destroy(&spinlock);
return 0;
}
```
在上面的代码中,我们使用了`pthread_spinlock_t`类型的变量`spinlock`来表示自旋锁。在`thread_func`函数中,我们首先使用`pthread_spin_lock`函数来获取自旋锁,然后对共享资源进行操作,最后使用`pthread_spin_unlock`函数释放自旋锁。在`main`函数中,我们创建了两个线程来同时访问共享资源。
需要注意的是,自旋锁只适用于多核处理器或者多线程并发执行的情况。如果在单核处理器上使用自旋锁,会导致线程一直处于忙等待状态,浪费CPU资源。
相关推荐
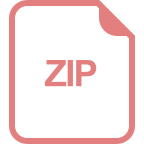
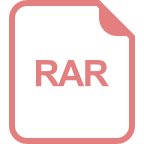
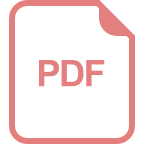















