c#提示无法从system.drawing.Rectangle转化为system.intptr
时间: 2024-12-28 16:37:08 浏览: 3
在 C# 中,`System.Drawing.Rectangle` 和 `System.IntPtr` 是两个不同的类型。Rectangle 是一个用于表示二维矩形区域的对象,包含左上角的 X 和 Y 坐标以及宽度和高度信息,它是结构类型。而 `IntPtr` 是一个整数类型的包装器,代表一个无符号长整型值,常用于内存地址的操作。
当试图将 `Rectangle` 类型转换为 `IntPtr` 时,因为它们的数据类型和用途完全不同,这种转换通常是不合法的,并且编译器会抛出错误。`Rectangle` 对象并不直接对应内存地址,所以不能简单地将其视为一个整数值。
如果确实需要将 `Rectangle` 的位置坐标(X、Y)转换为 `IntPtr`,你可以先提取坐标并单独转换,例如:
```csharp
Rectangle rect = ...; // 假设有一个矩形对象
int x = rect.X;
int y = rect.Y;
// 将坐标转换成 IntPtr
IntPtr intptr = new IntPtr((x << 16) + y); // 注意这里是将坐标打包成一个整数
```
这里我们假设 `X` 和 `Y` 都小于 `Int32.MaxValue`,以便能够通过位运算拼接。然而,通常这样的转换并不是必需的,除非有特殊的具体应用场景。
相关问题
C# System.Drawing.Image截取Rectangle范围的图像
在C#中,如果你想要从`System.Drawing.Image`对象中截取特定矩形区域的图像,你可以使用`GetThumbnailImage()`方法或者直接操作位图数据。以下是两种常见方法:
1. 使用`GetThumbnailImage()`方法:
```csharp
using System.Drawing;
// 假设你有一个名为image的Image对象
Rectangle rect = new Rectangle(x, y, width, height); // x, y, width, height是你要截取的区域坐标
Image croppedImage = image.GetThumbnailImage(rect.Width, rect.Height, null, IntPtr.Zero);
```
这里`GetThumbnailImage()`会生成一个新的缩略图图像,适合于小范围的截取。
2. 直接操作位图数据(Bitmap):
```csharp
using (Bitmap bitmap = new Bitmap(image))
{
using (Graphics graphics = Graphics.FromImage(bitmap))
{
graphics.DrawImage(image, new Rectangle(0, 0, bitmap.Width, bitmap.Height), rect.X, rect.Y, rect.Width, rect.Height, GraphicsUnit.Pixel);
// 现在bitmap变量就是截取后的图像
croppedImage = bitmap;
}
}
```
这个方法通过创建一个新的Bitmap并复制源图像的一部分到新Bitmap上,实现了精确的矩形截取。
system.drawing region 转换为opencv mat 区域
要将C#的System.Drawing.Region对象转换为OpenCV的Mat对象,你需要进行以下步骤:
1. 获取Region的边界矩形。
2. 创建一个Mat对象,大小与边界矩形相同。
3. 遍历Mat对象中的每个像素,根据像素是否在Region中来设置像素值。
以下是一个简单的示例代码:
```csharp
using System.Drawing;
using Emgu.CV;
using Emgu.CV.Structure;
// 获取Region的边界矩形
Rectangle rect = region.GetBounds(Graphics.FromHwnd(IntPtr.Zero));
// 创建Mat对象
Mat mat = new Mat(rect.Height, rect.Width, DepthType.Cv8U, 1);
// 遍历Mat对象中的每个像素
for (int y = 0; y < mat.Height; y++)
{
for (int x = 0; x < mat.Width; x++)
{
// 判断像素是否在Region中
if (region.IsVisible(new Point(x + rect.X, y + rect.Y)))
{
// 在Mat对象中设置像素值
mat.Data[y, x, 0] = 255;
}
else
{
mat.Data[y, x, 0] = 0;
}
}
}
```
在这个示例中,我们首先获取Region的边界矩形,然后创建一个大小与边界矩形相同的Mat对象。接着,我们遍历Mat对象中的每个像素,并根据像素是否在Region中来设置像素值。最后,我们得到了一个二值化的Mat对象,可以在OpenCV中进行进一步的处理。
阅读全文
相关推荐
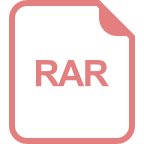
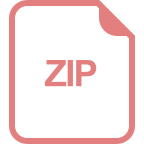
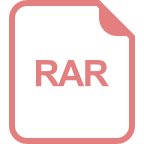













