java多线程 execute方法
时间: 2023-11-21 13:50:26 浏览: 37
Java多线程中的`execute`方法是不存在的,可能你想问的是`Executor`框架中的`execute`方法。这个方法用于提交一个`Runnable`任务给线程池执行。
例如:
```java
Executor executor = Executors.newFixedThreadPool(10);
executor.execute(new Runnable() {
@Override
public void run() {
// 线程执行的代码
}
});
```
这样就会将这个`Runnable`任务提交给线程池中的一个线程执行。
相关问题
java多线程执行方法
Java多线程实现的方式有四种:
1. 继承Thread类,重写run方法
```java
public class MyThread extends Thread {
public void run() {
// 线程执行的代码
}
}
MyThread myThread = new MyThread();
myThread.start(); // 启动线程
```
2. 实现Runnable接口,重写run方法,实现Runnable接口的实现类的实例对象作为Thread构造函数的target
```java
public class MyRunnable implements Runnable {
public void run() {
// 线程执行的代码
}
}
MyRunnable myRunnable = new MyRunnable();
Thread thread = new Thread(myRunnable);
thread.start(); // 启动线程
```
3. 通过Callable和FutureTask创建线程
```java
public class MyCallable implements Callable<String> {
public String call() throws Exception {
// 线程执行的代码
return "result";
}
}
MyCallable myCallable = new MyCallable();
FutureTask<String> futureTask = new FutureTask<>(myCallable);
Thread thread = new Thread(futureTask);
thread.start(); // 启动线程
String result = futureTask.get(); // 获取线程执行结果
```
4. 通过线程池创建线程
```java
ExecutorService executorService = Executors.newFixedThreadPool(10); // 创建线程池
executorService.execute(new Runnable() {
public void run() {
// 线程执行的代码
}
});
executorService.shutdown(); // 关闭线程池
```
java实现多线程的方法
Java实现多线程有以下几种方法:
1. 继承Thread类:创建一个继承自Thread类的子类,并重写其run()方法。然后通过创建子类对象并调用start()方法来启动线程。
```java
class MyThread extends Thread {
public void run() {
// 线程执行的代码
}
}
// 启动线程
MyThread thread = new MyThread();
thread.start();
```
2. 实现Runnable接口:创建一个实现了Runnable接口的类,并实现其run()方法。然后通过创建该类对象,并将其作为参数传递给Thread类的构造方法来创建线程对象。
```java
class MyRunnable implements Runnable {
public void run() {
// 线程执行的代码
}
}
// 启动线程
Thread thread = new Thread(new MyRunnable());
thread.start();
```
3. 使用Callable和Future:创建一个实现了Callable接口的类,并实现其call()方法。通过创建ExecutorService线程池,提交Callable任务并获取Future对象,最后通过Future对象获取任务的返回值。
```java
class MyCallable implements Callable<Integer> {
public Integer call() throws Exception {
// 线程执行的代码
return result;
}
}
// 创建线程池
ExecutorService executor = Executors.newFixedThreadPool(1);
// 提交Callable任务并获取Future对象
Future<Integer> future = executor.submit(new MyCallable());
// 获取任务的返回值
Integer result = future.get();
```
4. 使用线程池:创建一个线程池,通过调用execute()方法或submit()方法来提交任务,并由线程池自动管理线程的创建、销毁和复用。
```java
// 创建线程池
ExecutorService executor = Executors.newFixedThreadPool(5);
// 提交任务
executor.execute(new Runnable() {
public void run() {
// 线程执行的代码
}
});
```
以上是Java实现多线程的几种常见方法,根据不同的需求和场景选择适合的方式来实现多线程。
相关推荐
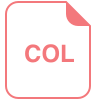
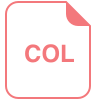
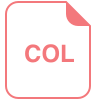
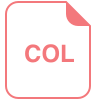
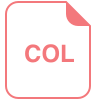










