在java中利用IOEception计算梯形面积
时间: 2024-09-09 21:06:05 浏览: 47
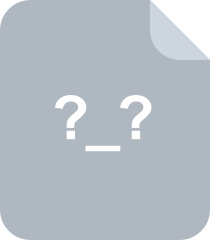
C++计算梯形面积.cpp
在Java中,IOException通常用于处理输入输出操作中的错误,比如文件读写、网络通信等异常情况。如果你想用它来计算梯形面积,那将是一个不合适的情境,因为IOException是用来处理I/O错误的,而不是数学计算。
然而,如果你确实想实现一个计算梯形面积的功能,并且希望处理可能出现的异常,例如除数为零导致的ArithmeticException,你可以这样做:
```java
import java.io.IOException; // 引入IO异常处理
public class TrapezoidArea {
public static double calculateTrapezoidArea(double base1, double base2, double height) {
try {
if (height <= 0) { // 检查基础条件,防止除以零
throw new ArithmeticException("Height cannot be zero or negative");
}
double area = (base1 + base2) * height / 2;
return area;
} catch (ArithmeticException e) { // 处理除法异常
System.out.println("Error: " + e.getMessage());
// 这里可以选择返回默认值,抛出异常,或者其他适当的错误处理方式
return 0;
} catch (IOException ioe) { // 处理IO异常,但这在这种场景下不会发生
System.out.println("An IO error occurred: " + ioe.getMessage());
// 同样,这里也需要适当地处理IO异常
}
}
public static void main(String[] args) {
double base1 = 5, base2 = 8, height = 4;
try {
double result = calculateTrapezoidArea(base1, base2, height);
System.out.printf("The area of the trapezoid is %.2f\n", result);
} catch (ArithmeticException e) {
// 主函数处理异常的情况
}
}
}
```
在这个例子中,`calculateTrapezoidArea`方法用于计算梯形面积,如果遇到`ArithmeticException`(如除以零),则捕获并处理这个异常。
阅读全文
相关推荐
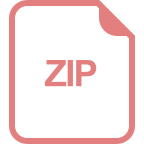
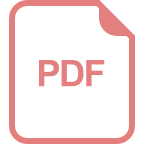
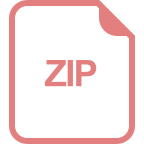
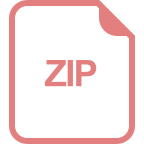
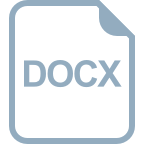
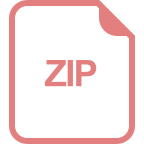
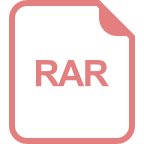
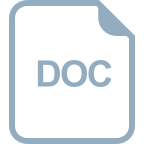
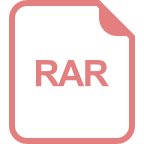
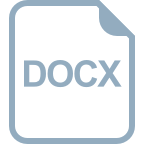