python实现将文字写到图片上
时间: 2024-10-01 09:12:51 浏览: 27
在Python中,可以使用`PIL`(Python Imaging Library)或者其升级版`Pillow`库来在图片上添加文字。以下是一个基本的例子,我们将使用`PIL`:
```python
from PIL import Image, ImageDraw, ImageFont
# 加载图片
img = Image.open('input.jpg')
# 创建一个Draw对象,在图像上绘图
draw = ImageDraw.Draw(img)
# 设置字体和大小
font = ImageFont.truetype("arial.ttf", 30) # 这里假设你有arial.ttf字体文件
# 定义文字和位置
text = "Hello, World!"
position = (10, 10) # x, y坐标,单位为像素
# 在图片上写入文字
draw.text(position, text, fill=(255, 255, 255), font=font) # 白色的文字,透明背景
# 保存修改后的图片
output_file = 'output_with_text.jpg'
img.save(output_file)
print(f"文字已添加到图片并保存为: {output_file}")
相关问题
python实现将文字写到图片上,保存并显示新图片
在Python中,你可以使用一些库如`PIL`(Python Imaging Library)或者更现代的`Pillow`、`ImageDraw`和`matplotlib`等来实现文字写入图片的功能。这里是一个基本的例子,我们将使用`PIL`:
```python
from PIL import Image, ImageDraw, ImageFont
# 创建一个空白的图片
img_width, img_height = 400, 200
image = Image.new('RGB', (img_width, img_height), color='white')
draw = ImageDraw.Draw(image)
# 设置字体和文本
font_path = 'arial.ttf' # 字体文件路径
font_size = 50
text = "Hello, World!"
# 加载字体
try:
font = ImageFont.truetype(font_path, font_size)
except IOError:
print("无法加载字体文件,请检查路径或替换为可用字体")
# 定义位置和颜色
text_position = (50, 75)
text_color = 'black'
# 写入文字
draw.text(text_position, text, fill=text_color, font=font)
# 保存图片
image.save('output_image.png')
# 显示图片
image.show()
Python实现图片文字识别
Python 实现图片文字识别通常涉及到光学字符识别(Optical Character Recognition, OCR),这是一项技术,用于从图像中提取出文本内容。以下是使用 Python 进行 OCR 的基本步骤:
1. **选择库**:最常用的 Python OCR 库包括 Tesseract, PyTesseract, PIL (Pillow) 和 OpenCV等。Tesseract 是一个开源的 OCR 工具,PyTesseract 是它的 Python 接口。
2. **安装依赖**:首先需要安装 pytesseract 和 Tesseract OCR 数据包。如果你使用的是 Ubuntu 或 Debian 系统,可以使用 `apt-get` 安装;如果是 Windows,可以从官网下载并配置环境变量。
3. **读取图片**:使用 Pillow 库打开图片文件,将图片转换为灰度或二值图,以便提高 OCR 效率。
```python
from PIL import Image
image = Image.open('example.jpg')
```
4. **预处理图像**:根据具体情况调整图像大小、去噪、对比度增强等,帮助 OCR 更好地识别文字。
5. **运行 OCR**:使用 pytesseract 对预处理后的图片进行文字识别,并获取识别结果。
```python
import pytesseract
text = pytesseract.image_to_string(image)
print(text)
```
6. **错误处理**:由于 OCR 技术的限制,识别结果可能存在误差,可能需要后期处理或结合其他算法进行校正。
注意:为了获得最佳效果,Tesseract 需要预先训练数据,特别是针对特定字体和语言的数据集。
阅读全文
相关推荐
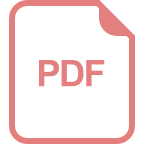
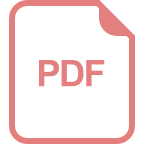
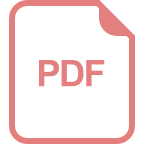
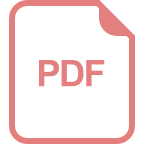
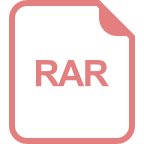
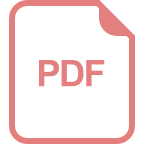
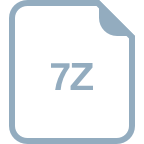
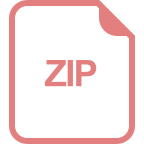
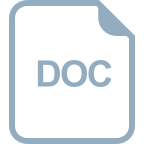
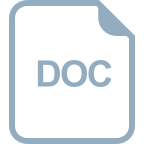
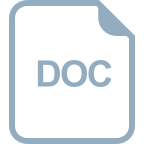
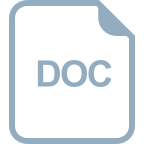
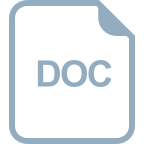
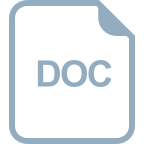

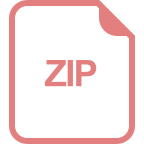