python实现读取图片中文字
时间: 2024-01-22 19:14:33 浏览: 28
要实现读取图片中的文字,可以使用Python中的OCR库,比如Tesseract或者PyOCR。
以下是使用PyOCR的示例代码:
```python
import sys
import pyocr
from PIL import Image
def recognize_text(image_path):
# 获取OCR工具
tools = pyocr.get_available_tools()
if len(tools) == 0:
print("No OCR tool found")
sys.exit(1)
tool = tools[0]
# 读取图片
image = Image.open(image_path)
# 识别文本
text = tool.image_to_string(
image,
lang='eng',
builder=pyocr.builders.TextBuilder()
)
return text
# 测试代码
if __name__ == '__main__':
image_path = 'test.png'
text = recognize_text(image_path)
print(text)
```
在上面的示例代码中,我们使用了PyOCR库来获取OCR工具,并使用该工具对指定的图片进行文本识别。最后输出识别出来的文本。
相关问题
python 如何实现识别图片中文字的位置
实现识别图片中文字的位置可以借助于 Python 中的 OCR(Optical Character Recognition,光学字符识别)技术,常用的 OCR 库有 Tesseract、OCRopus、pytesseract 等。下面以 pytesseract 库为例,介绍如何实现识别图片中文字的位置。
1. 安装 pytesseract 库
使用 pip 安装 pytesseract:
```
pip install pytesseract
```
2. 安装 Tesseract OCR 引擎
pytesseract 库是基于 Tesseract OCR 引擎开发的,因此需要先安装 Tesseract OCR 引擎。在安装 Tesseract OCR 引擎之前,需要先安装其依赖项。以 Ubuntu 为例,执行以下命令:
```
sudo apt-get update
sudo apt-get install libleptonica-dev libtesseract-dev tesseract-ocr
```
3. 实现识别图片中文字的位置
以下代码实现了从图片中识别出文字,并且获取文字的位置信息:
```python
import pytesseract
from PIL import Image
# 读取图片
image = Image.open('image.png')
# 识别文字
text = pytesseract.image_to_string(image)
# 获取文字区域
boxes = pytesseract.image_to_boxes(image)
# 打印识别结果和文字区域
print(text)
print(boxes)
```
其中 `image_to_string` 函数实现了识别图片中的文字,`image_to_boxes` 函数实现了获取文字区域。函数返回的 `boxes` 变量中包含了每个字符的位置信息,例如:
```
H 1 92 6 109 0
e 8 92 20 109 0
l 24 92 32 109 0
l 35 92 44 109 0
o 48 92 55 109 0
w 64 92 76 109 0
o 78 92 87 109 0
r 89 92 96 109 0
l 97 92 105 109 0
d 108 92 116 109 0
```
其中每行代表一个字符,以空格分隔的四个数分别表示字符、左下角 x 坐标、左下角 y 坐标、右上角 x 坐标、右上角 y 坐标。可以根据这些位置信息来获取文字的具体位置。
python svm读取中文图片
在 Python 中使用 SVM 对中文图片进行分类,需要经过以下几个步骤:
1. 导入 SVM 模型及相关库:
```python
from sklearn.svm import SVC
from sklearn.model_selection import train_test_split
import cv2
import numpy as np
```
2. 加载图片数据集:
```python
# 定义图片路径和标签文件路径
img_dir = 'path/to/image/directory/'
label_file = 'path/to/label/file.txt'
# 读取标签文件
with open(label_file, 'r') as f:
labels = f.read().splitlines()
# 读取图片并将其转换为灰度图像
images = []
for label in labels:
img_path = img_dir + label
img = cv2.imread(img_path, cv2.IMREAD_GRAYSCALE)
img = cv2.resize(img, (28, 28)) # 将图片大小调整为 28x28
images.append(img)
# 将图片数据转换为 NumPy 数组
images = np.array(images)
labels = np.array(labels)
```
3. 将数据集拆分为训练集和测试集:
```python
X_train, X_test, y_train, y_test = train_test_split(images, labels, test_size=0.3, random_state=42)
```
4. 将图片数据转换为 SVM 模型所需要的格式:
```python
# 将图片数据从二维数组转换为一维数组
X_train = X_train.reshape(X_train.shape[0], -1)
X_test = X_test.reshape(X_test.shape[0], -1)
# 将像素值归一化到 [0, 1] 的范围内
X_train = X_train.astype('float32') / 255.0
X_test = X_test.astype('float32') / 255.0
```
5. 定义 SVM 模型并进行训练:
```python
clf = SVC(kernel='linear')
clf.fit(X_train, y_train)
```
6. 使用测试集进行预测:
```python
y_pred = clf.predict(X_test)
```
7. 对预测结果进行评估:
```python
from sklearn.metrics import accuracy_score
acc = accuracy_score(y_test, y_pred)
print("Accuracy:", acc)
```
8. 读取中文图片:
由于 SVM 只能进行分类,无法直接输出图片的中文信息。如果需要实现中文图片识别,需要通过对中文图片进行 OCR(Optical Character Recognition 光学字符识别)处理,将图片中的文字转换为计算机可识别的文本数据。目前常用的 OCR 工具有 Tesseract、OCRopus 等,你可以根据自己的需求选择合适的工具进行学习和实践。
相关推荐
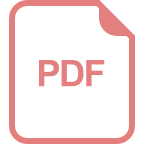
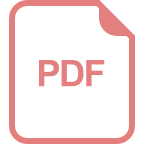
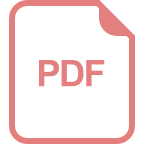












