用c语言写出:输出五天后的日期代码
时间: 2024-12-06 18:26:38 浏览: 4
在C语言中,你可以使用标准库中的`<time.h>`和`<stdio.h>`头文件来处理日期和时间。下面是一个简单的示例,它会获取当前日期并加5天后输出:
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
// 获取当前时间戳(秒)
time_t current_time = time(0);
// 加上5天
time_t future_time = current_time + (5 * 24 * 60 * 60); // 一天有86400秒
// 转换回struct tm格式的时间
struct tm* future_date = localtime(&future_time);
// 输出日期
printf("未来的五天日期: %d-%02d-%02d\n", future_date->tm_mday, future_date->tm_mon + 1, future_date->tm_year + 1900);
// 相关问题:
1. 这段代码为什么需要包含`localtime()`函数?
2. 如果我想让程序在用户输入的任意天数后显示日期,应该如何修改?
3. C语言中有没有内置的方式来直接表示日期,而不必先计算出时间戳?
相关问题
用C语言写出电子日历代码
以下是使用 C 语言编写的简单电子日历代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
int main() {
// 获取当前时间
time_t t = time(NULL);
struct tm *now = localtime(&t);
int year = now->tm_year + 1900; // 年份需要加上 1900
int month = now->tm_mon + 1; // 月份从 0 开始,需要加上 1
// 输出日历
printf(" %d年%d月\n", year, month);
printf("日 一 二 三 四 五 六\n");
struct tm date = {0, 0, 0, 1, month - 1, year - 1900}; // 初始日期为本月第一天
int weekday = mktime(&date) / 86400 % 7; // 计算本月第一天是星期几
for (int i = 0; i < weekday; i++) {
printf(" ");
}
for (int i = 1; i <= 31; i++) { // 一个月最多 31 天
date.tm_mday = i;
if (mktime(&date) / 86400 % 7 == 0 && i != 1) { // 如果是周日且不是本月第一天,则换行
printf("\n");
}
if (i > 9) {
printf("%d", i);
} else {
printf(" %d", i);
}
printf(" ");
if (mktime(&date) / 86400 % 7 == 6) { // 如果是周六,则换行
printf("\n");
}
}
return 0;
}
```
运行代码后,程序会自动获取当前年份和月份,并输出对应的日历。你可以根据需要对代码进行修改和扩展,以实现更多功能。
阅读全文
相关推荐
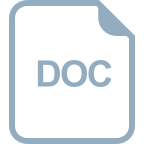
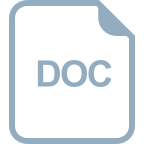
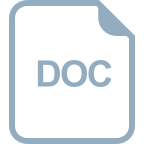
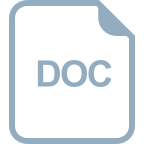
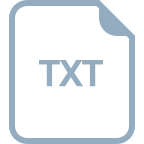
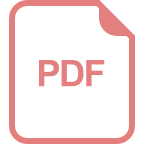
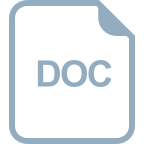
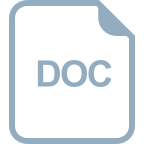
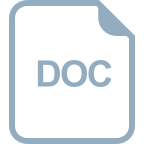
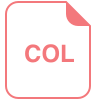
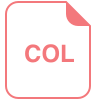
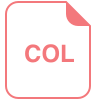
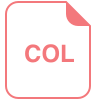
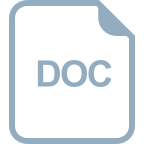
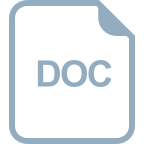
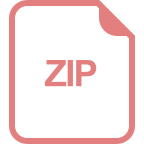
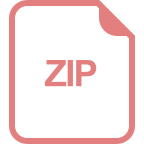
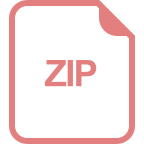