用c语言,一般来说,对于一个金融上的数字,我们每三个数加一个千分位分隔符,分别对应1,000(千),100,000(十万),100,000,000(千万)等等,在此,给定一个整型数字,要求输出在合适的地方加上千分位分隔符。
时间: 2024-09-25 12:17:15 浏览: 63
在C语言中,处理这种需求通常会涉及到字符串操作,因为我们需要动态地添加字符到字符串中。可以使用以下步骤实现:
1. 首先,创建一个空字符串`output`用于存放结果。
2. 定义一个辅助函数如`add_comma()`,用于在适当的位置插入逗号,并将剩余的数值转换成字符串。
3. 使用`itoa()`函数将整数转换成字符串,然后遍历每一位。
4. 当遇到整数的千位倍数(即除以1000余数为0)时,调用`add_comma()`并在其返回的字符串前添加千分位分隔符","。
5. 每次循环结束后,将当前部分追加到`output`。
6. 循环完成后,`output`字符串就是最终的结果。
这里是一个简单的示例代码片段:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
void add_comma(char* str, int len) {
char* temp = (char*)malloc(len + 2); // +2 for the comma and null terminator
if(temp == NULL)
return;
int i = len - 1;
while(i >= 0 && str[i] != '0') { // Move back from right until non-zero digit found
temp[i+1] = str[i];
i--;
}
temp[i+1] = '\0'; // Add null terminator at the end
if(i > 0) { // If there's a non-zero digit before the last three
temp[0] = str[i]; // Move that to the start
i++;
}
// Insert comma and append rest of the string
strcpy(&temp[i], ","); // Add comma
strcat(str, temp);
free(temp);
}
int main() {
int num;
printf("Enter an integer: ");
scanf("%d", &num);
char output[256];
add_comma(output, snprintf(output, sizeof(output), "%d", num)); // Convert and format number
printf("Formatted number: %s\n", output);
return 0;
}
```
阅读全文
相关推荐
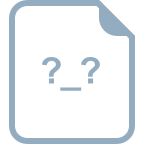
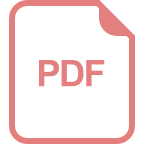
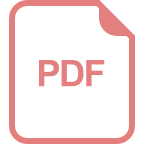
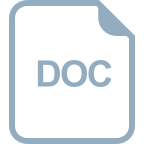





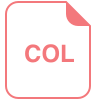








