WPF的rectangle做成一边圆角一边直角代码
时间: 2024-06-12 22:11:35 浏览: 151
在WPF中,可以通过自定义控件来实现一边圆角一边直角的矩形。以下是一个示例代码:
```
public class PartiallyRoundedRectangle : Shape
{
public static readonly DependencyProperty RadiusXProperty =
DependencyProperty.Register("RadiusX", typeof(double), typeof(PartiallyRoundedRectangle), new FrameworkPropertyMetadata(0.0, FrameworkPropertyMetadataOptions.AffectsRender));
public static readonly DependencyProperty RadiusYProperty =
DependencyProperty.Register("RadiusY", typeof(double), typeof(PartiallyRoundedRectangle), new FrameworkPropertyMetadata(0.0, FrameworkPropertyMetadataOptions.AffectsRender));
public static readonly DependencyProperty RoundTopLeftProperty =
DependencyProperty.Register("RoundTopLeft", typeof(bool), typeof(PartiallyRoundedRectangle), new FrameworkPropertyMetadata(false, FrameworkPropertyMetadataOptions.AffectsRender));
public static readonly DependencyProperty RoundTopRightProperty =
DependencyProperty.Register("RoundTopRight", typeof(bool), typeof(PartiallyRoundedRectangle), new FrameworkPropertyMetadata(false, FrameworkPropertyMetadataOptions.AffectsRender));
public static readonly DependencyProperty RoundBottomLeftProperty =
DependencyProperty.Register("RoundBottomLeft", typeof(bool), typeof(PartiallyRoundedRectangle), new FrameworkPropertyMetadata(false, FrameworkPropertyMetadataOptions.AffectsRender));
public static readonly DependencyProperty RoundBottomRightProperty =
DependencyProperty.Register("RoundBottomRight", typeof(bool), typeof(PartiallyRoundedRectangle), new FrameworkPropertyMetadata(false, FrameworkPropertyMetadataOptions.AffectsRender));
public double RadiusX
{
get { return (double)GetValue(RadiusXProperty); }
set { SetValue(RadiusXProperty, value); }
}
public double RadiusY
{
get { return (double)GetValue(RadiusYProperty); }
set { SetValue(RadiusYProperty, value); }
}
public bool RoundTopLeft
{
get { return (bool)GetValue(RoundTopLeftProperty); }
set { SetValue(RoundTopLeftProperty, value); }
}
public bool RoundTopRight
{
get { return (bool)GetValue(RoundTopRightProperty); }
set { SetValue(RoundTopRightProperty, value); }
}
public bool RoundBottomLeft
{
get { return (bool)GetValue(RoundBottomLeftProperty); }
set { SetValue(RoundBottomLeftProperty, value); }
}
public bool RoundBottomRight
{
get { return (bool)GetValue(RoundBottomRightProperty); }
set { SetValue(RoundBottomRightProperty, value); }
}
protected override Geometry DefiningGeometry
{
get
{
double width = RenderSize.Width;
double height = RenderSize.Height;
double radiusX = Math.Min(RadiusX, width / 2);
double radiusY = Math.Min(RadiusY, height / 2);
double topLeft = RoundTopLeft ? 1 : 0;
double topRight = RoundTopRight ? 1 : 0;
double bottomLeft = RoundBottomLeft ? 1 : 0;
double bottomRight = RoundBottomRight ? 1 : 0;
StreamGeometry geometry = new StreamGeometry();
using (StreamGeometryContext context = geometry.Open())
{
context.BeginFigure(new Point(radiusX, 0), true, true);
context.LineTo(new Point(width - radiusX, 0), true, false);
context.ArcTo(new Point(width, radiusY), new Size(radiusX, radiusY), 0, topRight == 1, SweepDirection.Clockwise, true, false);
context.LineTo(new Point(width, height - radiusY), true, false);
context.ArcTo(new Point(width - radiusX, height), new Size(radiusX, radiusY), 0, bottomRight == 1, SweepDirection.Clockwise, true, false);
context.LineTo(new Point(radiusX, height), true, false);
context.ArcTo(new Point(0, height - radiusY), new Size(radiusX, radiusY), 0, bottomLeft == 1, SweepDirection.Clockwise, true, false);
context.LineTo(new Point(0, radiusY), true, false);
context.ArcTo(new Point(radiusX, 0), new Size(radiusX, radiusY), 0, topLeft == 1, SweepDirection.Clockwise, true, false);
}
geometry.Freeze();
return geometry;
}
}
}
```
使用方法如下:
```
<local:PartiallyRoundedRectangle RadiusX="10" RadiusY="10" RoundTopLeft="True" Fill="LightBlue" Height="100" Width="200"/>
```
其中,RadiusX和RadiusY分别表示矩形的横向和纵向圆角半径,RoundTopLeft、RoundTopRight、RoundBottomLeft、RoundBottomRight分别表示矩形的四个角是否为圆角。
阅读全文
相关推荐
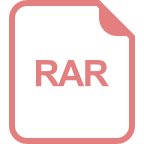
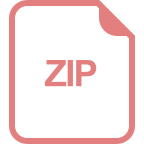
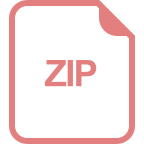















