spring boot 集成redis 请提供代码实现
时间: 2023-07-31 08:06:53 浏览: 84
当使用Spring Boot集成Redis时,可以使用Spring Data Redis来简化操作。下面是一个简单的代码示例:
1. 添加依赖:在`pom.xml`文件中添加`spring-boot-starter-data-redis`依赖。
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-redis</artifactId>
</dependency>
```
2. 配置Redis连接:在`application.properties`(或`application.yml`)文件中配置Redis连接信息。
```properties
spring.redis.host=localhost
spring.redis.port=6379
```
3. 创建Redis配置类:创建一个配置类,用于配置Redis连接工厂和Redis模板。
```java
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.data.redis.connection.RedisConnectionFactory;
import org.springframework.data.redis.connection.lettuce.LettuceConnectionFactory;
import org.springframework.data.redis.core.RedisTemplate;
import org.springframework.data.redis.serializer.StringRedisSerializer;
@Configuration
public class RedisConfig {
@Bean
public RedisConnectionFactory redisConnectionFactory() {
return new LettuceConnectionFactory();
}
@Bean
public RedisTemplate<String, String> redisTemplate() {
RedisTemplate<String, String> redisTemplate = new RedisTemplate<>();
redisTemplate.setConnectionFactory(redisConnectionFactory());
redisTemplate.setKeySerializer(new StringRedisSerializer());
redisTemplate.setValueSerializer(new StringRedisSerializer());
return redisTemplate;
}
}
```
4. 使用Redis操作:在需要使用Redis的地方,注入`RedisTemplate`对象即可进行操作。
```java
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.data.redis.core.RedisTemplate;
import org.springframework.stereotype.Service;
@Service
public class RedisService {
@Autowired
private RedisTemplate<String, String> redisTemplate;
public void setValue(String key, String value) {
redisTemplate.opsForValue().set(key, value);
}
public String getValue(String key) {
return redisTemplate.opsForValue().get(key);
}
}
```
在上述示例中,我们通过`RedisTemplate`对象进行了简单的set和get操作。你可以根据具体需求使用不同的Redis操作方法,例如`opsForHash()`用于操作哈希表、`opsForList()`用于操作列表等。
希望这个示例能帮助到你!如果还有其他问题,请随时提问。
相关推荐
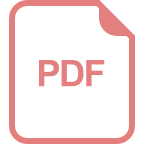
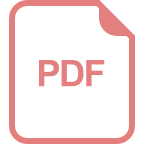














