vue中如何调用其他组件的方法
时间: 2023-10-13 14:45:43 浏览: 127
在Vue中,可以通过使用`$refs`来调用其他组件的方法。`$refs`是一个特殊的属性,可以用来访问组件实例或DOM元素。
首先,在要调用其他组件方法的组件中,给该组件添加一个`ref`属性,例如:
```html
<template>
<div>
<other-component ref="other"></other-component>
</div>
</template>
```
然后,在组件的方法中,使用`$refs`来访问该组件的实例,并调用其方法,例如:
```javascript
export default {
methods: {
callOtherComponentMethod() {
this.$refs.other.methodName();
}
}
}
```
这样,就可以通过`this.$refs.other`来访问`other-component`组件的实例,然后调用其方法`methodName()`。
需要注意的是,`$refs`只在组件渲染完成后才能访问到正确的实例,所以最好在`mounted`钩子函数或之后的生命周期钩子函数中使用`$refs`。
相关问题
vue中父组件调用子组件方法
在Vue中,父组件可以通过使用`ref`来获取子组件的实例,并调用子组件的方法。以下是一个简单的示例:
```vue
<template>
<div>
<ChildComponent ref="childComponent"></ChildComponent>
<button @click="callChildMethod">调用子组件方法</button>
</div>
</template>
<script>
import ChildComponent from './ChildComponent.vue';
export default {
components: {
ChildComponent,
},
methods: {
callChildMethod() {
this.$refs.childComponent.childMethod();
},
},
};
</script>
```
在上面的示例中,父组件通过在`ChildComponent`上添加`ref`属性来引用子组件实例。然后,通过`this.$refs.childComponent`可以访问子组件的实例,并调用子组件的方法`childMethod()`。
请注意,为了能够使用`ref`引用子组件,你需要确保在父组件中正确地声明了子组件,即在`components`选项中注册了子组件。
vue3调用父组件中的方法
在Vue3中,要调用父组件中的方法,可以通过以下步骤完成:
1. 首先,在子组件中注册一个事件。你可以在需要调用父组件方法的地方,使用`$emit`方法触发一个自定义事件,并传递参数给父组件。
2. 其次,在父组件中引入子组件,并且在子组件标签上使用`@`符号加上注册的事件名,将其绑定到父组件的一个方法上。这样当子组件触发了这个事件时,父组件中的方法就会被调用。
具体代码实现如下所示:
在子组件中注册事件:
```javascript
// 子组件
<template>
<button @click="$emit('custom-event', data)">触发事件</button>
</template>
<script>
export default {
data() {
return {
data: '需要传递给父组件的数据',
};
},
};
</script>
```
在父组件中调用子组件方法:
```javascript
// 父组件
<template>
<div>
<child-component @custom-event="handleCustomEvent"></child-component>
</div>
</template>
<script>
import ChildComponent from './ChildComponent.vue';
export default {
components: {
ChildComponent,
},
methods: {
handleCustomEvent(data) {
// 在这里可以调用父组件的方法,并且可以使用子组件传递过来的数据
},
},
};
</script>
```
通过以上步骤,你就可以在Vue3中成功调用父组件中的方法了。
阅读全文
相关推荐
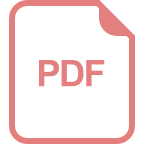
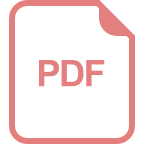
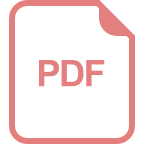












