c语言l利用栈判断字符串是否为回文串
时间: 2024-10-25 11:15:12 浏览: 25
在C语言中,你可以通过使用两个指针分别从字符串的首尾开始遍历,并将字符逐个入栈,然后依次出栈进行比较,来判断一个字符串是否是回文串。以下是基本步骤:
1. 定义两个指针,一个指向字符串的起始位置(start),另一个指向字符串的结束位置(end)。
2. 创建一个空栈,用于存放中间字符。
3. 当`start`小于等于`end`时,循环执行以下操作:
a. 将当前`start`位置的字符压入栈中。
b. 将`start`向右移动一位(`start++`)。
c. 如果`start`仍然小于或等于`end`,取出栈顶元素并与`end`位置的字符对比,如果不同则不是回文串,返回false。
d. 将`end`向左移动一位(`end--`)。
4. 遍历结束后,如果没有发现不匹配的字符,则整个字符串是回文串,返回true。
```c
#include <stdio.h>
#include <stdbool.h>
#include <string.h>
#include <stack>
bool isPalindrome(char str[]) {
stack<char> s;
int start = 0, end = strlen(str) - 1;
// 入栈
while (start <= end) {
s.push(str[start]);
start++;
}
// 出栈并对比
while (!s.empty()) {
if (str[end] != s.top()) {
return false;
}
s.pop();
end--;
}
return true;
}
int main() {
char test[] = "madam";
if (isPalindrome(test)) {
printf("%s 是回文串\n", test);
} else {
printf("%s 不是回文串\n", test);
}
return 0;
}
```
阅读全文
相关推荐
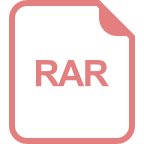
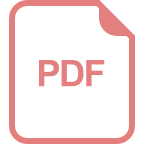






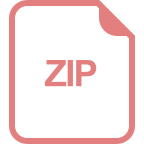







