用c语言写一个判断字符串是否为回文的函数
时间: 2024-11-15 17:24:16 浏览: 9
在 C 语言中,你可以创建一个名为 `is_palindrome()` 的函数来判断输入的字符串是否是回文。由于 C 语言没有内置的字符串类型,我们可以使用数组来代表字符串,并遍历数组从两端同时检查字符是否相等。下面是一个简单的示例:
```c
#include <stdio.h>
#include <stdbool.h>
#include <string.h> // 包含 strlen 函数
bool is_palindrome(char* str) {
int len = strlen(str); // 获取字符串长度
int start = 0;
int end = len - 1;
while (start < end) { // 双指针法
if (str[start] != str[end]) { // 比较当前字符
return false; // 非回文
}
start++; // 向前移动
end--; // 后退移动
}
return true; // 所有字符匹配,是回文
}
// 测试函数
int main() {
char test_str[] = "madam";
printf("%s is a palindrome? %d\n", test_str, is_palindrome(test_str));
return 0;
}
```
这个函数接收一个字符数组作为输入,通过逐个比较首尾字符并逐渐向中间移动,来判断整个字符串是否为回文。
阅读全文
相关推荐
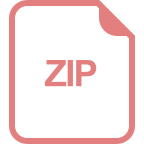
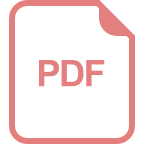
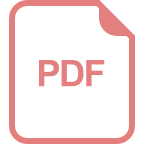















