用C语言编写求排列数的函数
时间: 2024-10-29 22:10:17 浏览: 7
在C语言中,计算排列数(Permutations)通常涉及到递归或者利用阶乘的概念。排列数对于n个不同元素来说是 n! (n 的阶乘),即所有从1到n的整数相乘的结果。下面是一个简单的C函数,使用了递归来计算阶乘并进而得到排列数:
```c
#include <stdio.h>
// 计算阶乘的辅助函数
int factorial(int n) {
if (n == 0 || n == 1) {
return 1;
}
else {
return n * factorial(n - 1);
}
}
// 求排列数的函数
int permutation(int n) {
return factorial(n);
}
int main() {
int num_elements;
printf("Enter the number of elements: ");
scanf("%d", &num_elements);
if(num_elements < 0)
printf("Error: Number of elements cannot be negative.\n");
else {
long long result = permutation(num_elements);
printf("The number of permutations for %d elements is %lld\n", num_elements, result);
}
return 0;
}
```
在这个例子中,`factorial()`函数负责计算阶乘,然后`permutation()`函数直接返回这个结果。注意,由于阶乘会增长得非常快,当n较大时可能会超出int类型的范围,所以这里使用long long来存储结果。
阅读全文
相关推荐
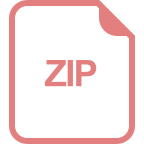
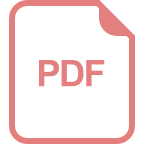
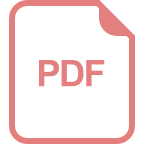
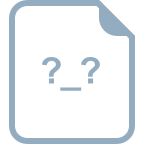














