使用Java的switch语句编写程序输入某年某月,输出该月多少天
时间: 2024-12-17 22:36:22 浏览: 8
在Java中,你可以使用`switch`语句配合常量枚举来编写这样的程序。首先,你需要创建一个枚举类型,代表每个月份的天数,因为并不是所有月份都有相同的天数。然后,通过用户输入的年份和月份,判断并输出对应的天数。
以下是一个简单的示例:
```java
import java.util.Scanner;
public class MonthDays {
enum DaysInMonth {
FEBRUARY(28), MARCH(31), APRIL(30), MAY(31),
JUNE(30), JULY(31), AUGUST(31), SEPTEMBER(30),
OCTOBER(31), NOVEMBER(30), DECEMBER(31);
private int days;
DaysInMonth(int days) {
this.days = days;
}
public int getDays() {
return days;
}
}
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("请输入年份:");
int year = scanner.nextInt();
System.out.println("请输入月份(1-12):");
int month = scanner.nextInt();
switch (month) {
case 1:
case 3:
case 5:
case 7:
case 8:
case 10:
case 12:
// 除了二月,其他都是31天
System.out.printf("在%d年%d月,有%d天.\n", year, month, DaysInMonth.JULY.getDays());
break;
case 4:
case 6:
case 9:
case 11:
// 四季的月份都是30天
System.out.printf("在%d年%d月,有%d天.\n", year, month, DaysInMonth.AUGUST.getDays());
break;
case 2:
// 如果是闰年的二月,则29天;非闰年则28天
if (isLeapYear(year)) {
System.out.printf("在%d年闰年的%d月,有%d天.\n", year, month, DaysInMonth.FEBRUARY.getDays());
} else {
System.out.printf("在%d年非闰年的%d月,有%d天.\n", year, month, DaysInMonth.FEBRUARY.getDays());
}
break;
default:
System.out.println("无效的月份");
break;
}
scanner.close();
}
// 判断是否为闰年,这里简化处理,只考虑能被4整除但不能被100整除,或者可以被400整除的情况
private static boolean isLeapYear(int year) {
return (year % 4 == 0 && year % 100 != 0) || (year % 400 == 0);
}
}
```
在这个例子中,`switch`语句根据月份的输入选择相应的`DaysInMonth`常量,获取对应的天数。如果需要更精确地判断平闰年,还需要额外的闰年检查函数。
阅读全文
相关推荐
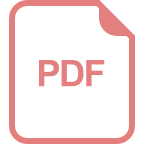
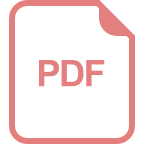
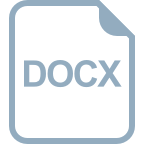

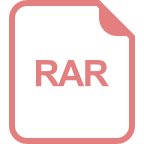
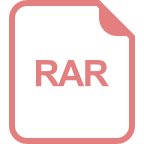
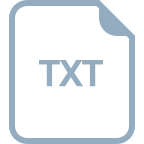
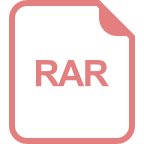
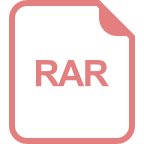
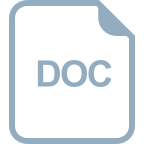
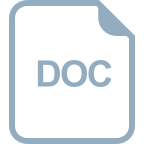
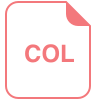
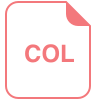





