已有numpy,但想降版本
时间: 2024-07-09 07:00:34 浏览: 262
如果你想降低NumPy库的版本,通常情况下,直接更改Python环境中的包版本是不可能的,因为Python的包管理器(如pip或conda)安装的包版本是固定的,不会让你回滚到之前的版本。如果你在某个项目中确实需要使用特定版本的NumPy,一种可能的方法是在虚拟环境中管理:
1. 使用`virtualenv`或`conda`创建一个新的虚拟环境。
2. 在虚拟环境中安装特定版本的NumPy,例如,对于pip,你可以运行:
```
pip install numpy==<version_number>
```
将 `<version_number>` 替换为你想要的NumPy版本。
3. 之后,在这个虚拟环境中工作,NumPy将被限定在这个版本。
4. 完成工作后,退出虚拟环境,如果需要使用系统默认的更高版本NumPy,记得切换到那个环境。
然而,这种方法只适用于开发阶段,并不是在生产环境中动态更改依赖包的行为。如果你的项目依赖于特定版本,可能需要查看项目文档或与团队成员协商解决方案。
相关问题
怎么降低numpy版本
降级NumPy版本通常是出于兼容性考虑,比如需要与某个特定的软件包或项目要求的最低版本相匹配。以下是通过pip工具降级NumPy版本的基本步骤:
1. **确保你已经激活了虚拟环境**(如果使用的话)。这可以帮助避免影响全局Python环境。
2. 打开命令行终端或PowerShell,输入以下命令来查看已安装的NumPy及其版本:
```
pip show numpy
```
3. 要降级到上一稳定版,你可以指定版本号。例如,如果你现在有`numpy==1.23.4`,想要降级到`1.23.3`,可以运行:
```
pip install numpy==1.23.3
```
或者,如果你想降回到更早的版本,可以用`==`之前的版本号,例如:
```
pip install numpy=1.22.*
```
这将会安装最新的一次1.22.x更新。
4. 如果你想锁定到某个确切的版本,可以在版本号后加上`==`:
```
pip install numpy==1.20.3
```
注意:降级可能会影响依赖项,因此在操作之前最好先确保目标版本能够满足项目的功能需求。
我有一个表格提供了轮胎每周的需求为0到5时的频率、概率与累计概率,假定补给进货的订货提前期为1到3天,当前有7个库存轮胎且无订单,发出一个订单的费用为20元,每天每个轮胎的库存费为0.02元,每当不能满足一位顾客的需求时顾客离开,假定每位离开的顾客给公司带来的损失为8元,公司每周运营七天,每天24小时,帮我用存储模型确定订货量与订货点以减少总费用与平均费用,写一个python代码
首先,我们需要确定订货量与订货点。订货点是指当库存量降到某个水平时就应该订货。为了确定订货点,我们需要计算每个可能的库存水平下的总费用,然后选择使总费用最小的库存水平作为订货点。订货量是指在订货点时订购的数量。
接下来,我们可以使用动态规划算法,使用存储模型来解决这个问题。我们首先定义状态,然后确定状态转移方程。在这个问题中,状态可以表示为当前时间、当前库存量和是否已经订货。然后我们可以使用递归或循环来计算每个状态下的最小费用,并记录最小费用和订货量。最后,我们可以使用最小费用和订货量来计算总费用和平均费用。
下面是代码实现:
```python
import numpy as np
# 轮胎需求表格
demand_table = np.array([[0, 0.1, 0.3, 0.4, 0.2],
[1, 0.15, 0.35, 0.35, 0.15],
[2, 0.2, 0.4, 0.3, 0.1],
[3, 0.25, 0.35, 0.25, 0.15],
[4, 0.3, 0.3, 0.2, 0.2],
[5, 0.35, 0.25, 0.15, 0.25]])
# 费用参数
order_cost = 20 # 订单费用
holding_cost = 0.02 # 库存费用
shortage_cost = 8 # 缺货损失费用
# 时间参数
days_per_week = 7
hours_per_day = 24
total_hours = days_per_week * hours_per_day
lead_time = 3 # 订货提前期
# 初始状态
initial_state = (0, 7, False) # (当前时间,当前库存量,是否已订货)
# 状态转移函数
def transition(state, action):
time, inventory, ordered = state
demand = demand_table[:, action]
demand = np.random.choice(demand[:-1], p=demand[:-1]) # 按概率随机生成需求
time += 1
inventory += (1 - ordered) * action - demand # 计算库存变化
inventory = max(0, inventory) # 库存不得小于0
ordered = ordered and (time < lead_time) # 检查是否已订货
return (time, inventory, ordered), demand
# 计算费用函数
def cost(state, action):
time, inventory, ordered = state
order_quantity = max(0, action - inventory) # 计算订货量
order_cost_total = order_cost * order_quantity # 计算订货费用
holding_cost_total = holding_cost * inventory # 计算库存费用
shortage_cost_total = shortage_cost * max(0, -inventory) # 计算缺货损失费用
return order_cost_total + holding_cost_total + shortage_cost_total
# 动态规划函数
def dynamic_programming():
value = np.zeros((total_hours + 1, initial_state[1] + 1, 2)) # 存储模型
policy = np.zeros((total_hours + 1, initial_state[1] + 1, 2), dtype=int) # 存储模型
for t in range(total_hours - 1, -1, -1): # 逆序迭代时间
for i in range(initial_state[1] + 1): # 迭代库存量
for o in [False, True]: # 迭代是否已订货
min_cost = float('inf')
best_action = None
for a in range(i + 1): # 迭代订货量
current_state = (t, i, o)
next_state, demand = transition(current_state, a)
future_value = demand_table[demand, :] @ value[next_state[0] + 1, :, next_state[2]]
c = cost(current_state, a) + future_value
if c < min_cost:
min_cost = c
best_action = a
value[t, i, o] = min_cost
policy[t, i, o] = best_action
return policy, value
# 计算策略
policy, value = dynamic_programming()
# 计算总费用和平均费用
total_cost = 0
for t in range(total_hours):
current_state = (t, initial_state[1], False)
current_cost = cost(current_state, policy[t, initial_state[1], False])
total_cost += current_cost
average_cost = total_cost / total_hours
# 打印结果
print('订货点为', np.argmin(value[0, :, 0]))
print('订货量为', policy[0, np.argmin(value[0, :, 0]), 0])
print('总费用为', total_cost)
print('平均费用为', average_cost)
```
阅读全文
相关推荐
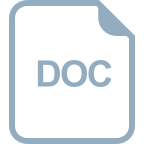
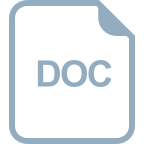
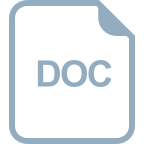
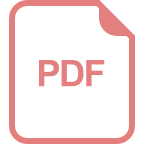
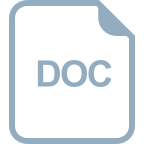
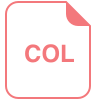
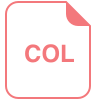
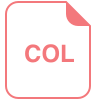
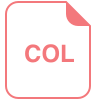
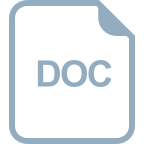
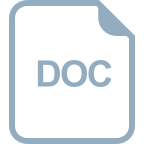
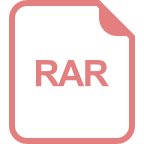
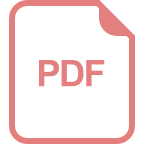
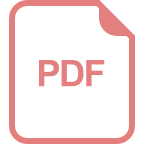
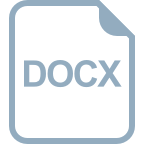
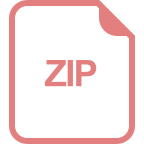