C语言基于栈的括号匹配的代码
时间: 2024-10-11 15:15:28 浏览: 38
C语言中基于栈的数据结构可以用来实现括号匹配的问题。这个问题通常通过递归深度优先搜索(DFS)或广度优先搜索(BFS)结合栈来解决。下面是一个简单的基于栈的递归函数示例:
```c
#include <stdbool.h>
#include <stdio.h>
#include <stdlib.h>
#define MAX_STACK_SIZE 50
bool isBalanced(char* str) {
stack<char> s;
int i = 0;
while (str[i] != '\0') {
if (str[i] == '(' || str[i] == '{' || str[i] == '[') { // 入栈左括号
s.push(str[i]);
} else if (str[i] == ')' || str[i] == '}' || str[i] == ']') { // 遇到右括号
char top = s.top();
s.pop();
// 如果当前右括号和栈顶左括号不匹配,则返回false
if ((str[i] == ')' && top != '(') ||
(str[i] == ']' && top != '[') ||
(str[i] == '}' && top != '{')) {
return false;
}
}
i++;
}
// 如果遍历完字符串还有剩余的左括号在栈里,说明不匹配
return s.empty() ? true : false;
}
int main() {
char str[] = "((()))";
if (isBalanced(str)) {
printf("The parentheses are balanced.\n");
} else {
printf("The parentheses are not balanced.\n");
}
return 0;
}
```
在这个例子中,`isBalanced` 函数使用了一个字符类型的栈 `s` 来跟踪遇到的左括号,当遇到右括号时,会检查栈顶的左括号是否匹配。如果栈为空或者栈顶的左括号不匹配,函数将返回 `false`,表示括号不匹配。
阅读全文
相关推荐
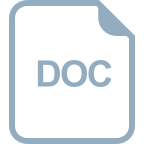
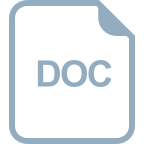
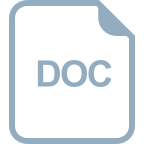
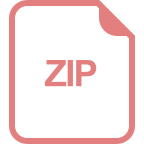
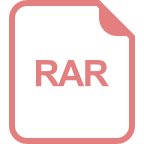
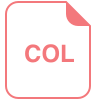
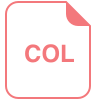





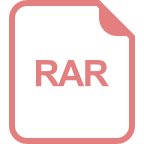
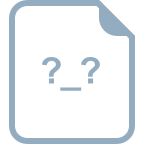
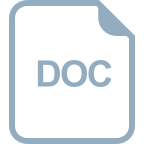
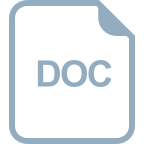
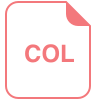
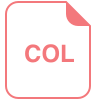
