通过链式存储与顺序存储分别实现括号匹配,并且可以通过菜单选择的c语言代码
时间: 2024-11-09 16:27:01 浏览: 22
在C语言中,我们可以分别使用链式存储(如利用栈的数据结构)和顺序存储(如数组)来实现括号匹配。这里我将提供一个简单的示例代码,首先是链式存储(基于栈),然后是顺序存储(遍历数组)。
**1. 链式存储 (栈实现)**
```c
#include <stdio.h>
#include <stdbool.h>
#define MAX 100
typedef struct Node {
char ch;
bool visited;
} Node;
bool isParenthesesMatch(char* str, Stack* stack) {
stack = initStack(stack);
for (int i = 0; str[i] != '\0'; ++i) {
if (str[i] == '(' || str[i] == '[' || str[i] == '{') {
push(str[i], stack);
} else if (str[i] == ')' || str[i] == ']' || str[i] == '}') {
if (stack->top == -1 || !matches(str[i], stack->top)) {
return false;
}
pop(stack);
}
}
return stack->top == -1; // 如果栈为空,则表示所有括号都已匹配
}
// ...其他函数(例如初始化栈、入栈、出栈等)
```
**2. 顺序存储 (数组实现)**
```c
#include <stdbool.h>
bool parenthesesMatch(char arr[], int n) {
bool match[n][n]; // 初始化一个二维布尔数组,用于记录对应位置的括号是否匹配
int openingBrackets[3] = {'(', '[', '{'}; // 开始标记
int closingBrackets[3] = {')', ']', '}'}; // 结束标记
int openIndex = 0;
for (int i = 0; i < n; ++i) {
if (arr[i] == openingBrackets[openIndex]) {
match[i][i] = true; // 自己配对
openIndex++;
} else {
int j;
for (j = i; j >= 0 && match[j][i] == false; --j) {
if (closingBrackets[openIndex] == arr[j]) {
match[j][i] = true;
break;
}
}
if (j == i) {
return false; // 未找到匹配
}
}
}
return true;
}
```
阅读全文
相关推荐
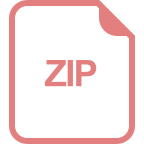
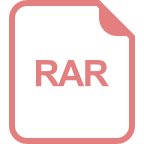
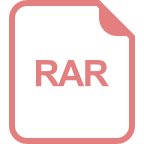

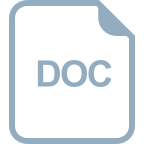
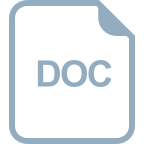
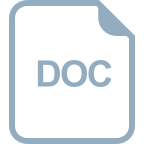
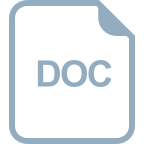
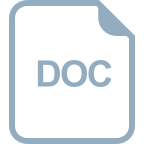
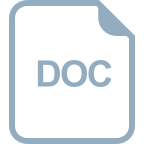
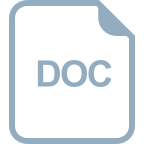
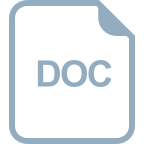
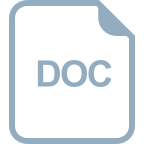
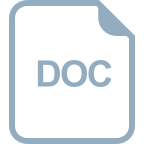
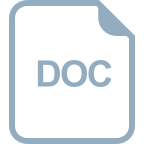
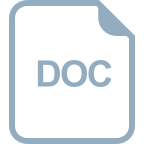