用C语言统计一个字符串中英文字母的个数。
时间: 2024-12-24 16:30:20 浏览: 5
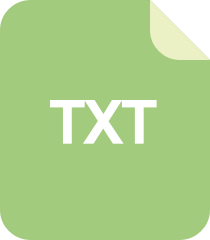
C语言实现打字练习程序

在C语言中,统计一个字符串中英文字母的个数,你可以使用`ctype.h`库提供的函数来判断字符是否为字母。以下是实现的步骤:
1. 包含头文件`ctype.h`,它包含了字符分类函数。
```c
#include <ctype.h>
```
2. 初始化两个计数器,分别用于英文大写字母和小写字母的个数。
```c
int upper_count = 0, lower_count = 0;
```
3. 遍历字符串中的每一个字符,对字母进行计数。
```c
for (int i = 0; string[i] != '\0'; i++) {
if (isalpha(string[i])) { // 判断字符是否为字母
if (isupper(string[i])) { // 英文大写字母
upper_count++;
} else if (islower(string[i])) { // 英文小写字母
lower_count++;
}
}
}
```
4. 最后返回总字母数(大小写合并)。
```c
int total_alphabets = upper_count + lower_count;
```
完整的代码示例:
```c
#include <ctype.h>
#include <stdio.h>
#include <string.h>
int count_letters(const char* string) {
int upper_count = 0, lower_count = 0;
for (int i = 0; string[i] != '\0'; i++) {
if (isalpha(string[i])) {
if (isupper(string[i])) {
upper_count++;
} else if (islower(string[i])) {
lower_count++;
}
}
}
int total_alphabets = upper_count + lower_count;
return total_alphabets;
}
int main() {
const char* test_string = "Hello, World!";
int alphabet_count = count_letters(test_string);
printf("字符串 '%s' 中英文字母的总数为: %d\n", test_string, alphabet_count);
return 0;
}
```
阅读全文
相关推荐
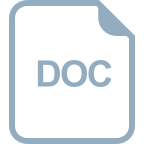
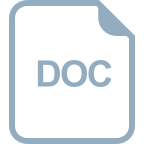















