根据输入的字符串智能识别出对应的货物名词和数量并且根据对应货物的单价完成计算
时间: 2024-04-19 18:24:15 浏览: 115
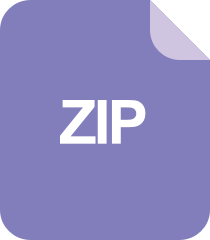
商品查找,单价计算
好的,以下是一个根据输入字符串智能识别货物名词和数量,并根据对应货物的单价完成计算的示例代码:
```java
import java.util.HashMap;
import java.util.Map;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
public class PriceCalculator {
public static void main(String[] args) {
String input = "豆干 5斤, 豆筋 5斤, 豆皮 5斤, 素鸡 4个, 河粉 10斤";
// 创建货物数量和单价的映射关系
Map<String, Double> priceMap = new HashMap<>();
priceMap.put("豆干", 10.0);
priceMap.put("豆筋", 8.0);
priceMap.put("豆皮", 6.0);
priceMap.put("素鸡", 15.0);
priceMap.put("河粉", 5.0);
// 添加更多货物的单价
// 使用正则表达式匹配货物名称和数量
Pattern pattern = Pattern.compile("(\\D+)(\\d+)(\\D+)");
Matcher matcher = pattern.matcher(input);
// 解析匹配结果并进行计算
double total = 0.0;
while (matcher.find()) {
String goods = matcher.group(1).trim();
int quantity = Integer.parseInt(matcher.group(2));
double price = priceMap.getOrDefault(goods, 0.0);
double itemTotal = price * quantity;
total += itemTotal;
System.out.println("货物名称:" + goods);
System.out.println("数量:" + quantity);
System.out.println("单价:" + price);
System.out.println("总价:" + itemTotal);
System.out.println();
}
System.out.println("总价格:" + total);
}
}
```
你可以将上述代码保存为一个名为 `PriceCalculator.java` 的文件并运行它。代码会根据输入的字符串智能识别出货物名称和数量,并根据对应货物的单价进行计算,输出每个货物的详细信息以及总价格。请注意,以上只是一个简单的示例代码,你可以根据实际需求进行修改和扩展。
阅读全文
相关推荐
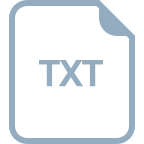
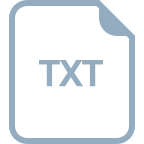
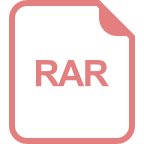
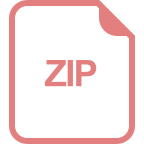
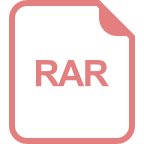
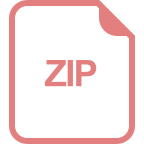
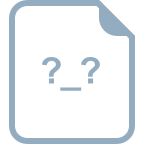
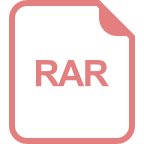
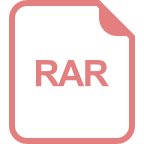
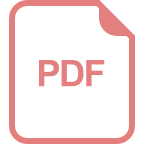
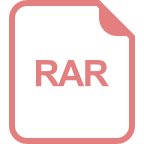
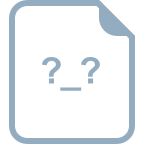