用python语言编程实现生产者-消费者问题
时间: 2024-10-25 18:08:31 浏览: 14
在Python中,生产者-消费者问题是一种经典的问题,通常用于演示并发处理和线程同步的概念。这个问题涉及到两个角色:生产者,负责生成数据;消费者,负责消费这些数据。为了保证数据安全,生产者和消费者需要共享一个队列,并且在操作队列之前需要遵守适当的锁机制。
以下是一个简单的基于`threading`模块实现的生产者-消费者模型:
```python
import queue
import threading
# 定义生产者线程
class Producer(threading.Thread):
def __init__(self, queue):
threading.Thread.__init__(self)
self.queue = queue
def run(self):
for i in range(5): # 生产5个元素
item = f"Producer produced item {i}"
print(f"{threading.current_thread().name} produced: {item}")
self.queue.put(item)
time.sleep(1) # 模拟生产时间
# 定义消费者线程
class Consumer(threading.Thread):
def __init__(self, queue):
threading.Thread.__init__(self)
self.queue = queue
def run(self):
while True:
item = self.queue.get() # 获取队列中的元素
if item is None: # 如果队列为空或None(表示生产者结束)
break
print(f"{threading.current_thread().name} consumed: {item}")
self.queue.task_done()
# 创建队列和线程
queue = queue.Queue()
producer = Producer(queue)
consumer = Consumer(queue)
# 启动线程
producer.start()
consumer.start()
# 等待生产者完成
producer.join()
# 设置信号给消费者,告诉他们生产者已停止
queue.put(None)
# 关闭消费者线程
consumer.join()
阅读全文
相关推荐
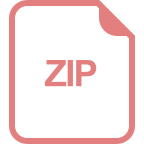
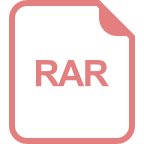
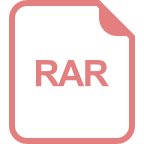

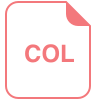
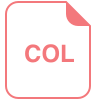
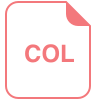


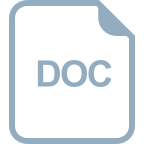
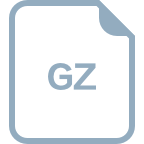
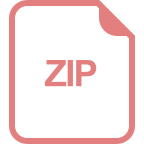
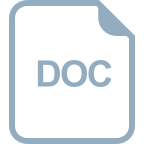
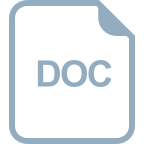
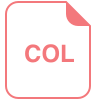

