用c语言编写一个程序,将一个字符串中的元音字母(a、e、i、o、u)复制到另一个字符串,然后按照ASCII码从小到大的顺序输出。要求运用数组。
时间: 2024-12-20 10:31:33 浏览: 24
下面是一个简单的C语言程序,它会读取一个字符串,找出其中的所有元音字母并将其复制到另一个字符串,最后按照ASCII码值排序并输出。
```c
#include <stdio.h>
#include <string.h>
// 定义元音字母数组
const char vowels[] = {'a', 'e', 'i', 'o', 'u', '\0'};
char input[100];
char output[50];
void copy_vowels(char* src, char* dst) {
int i;
for (i = 0; src[i] != '\0'; ++i) {
if (strchr(vowels, src[i]) != NULL) {
dst[i] = src[i];
}
}
dst[i] = '\0'; // 添加字符串结束符
}
void sort_and_output(char* arr, int len) {
int i, j;
for (i = 0; i < len - 1; ++i) {
for (j = 0; j < len - 1 - i; ++j) {
if (arr[j] > arr[j + 1]) { // 使用ASCII码比较
char temp = arr[j];
arr[j] = arr[j + 1];
arr[j + 1] = temp;
}
}
}
printf("Sorted vowels in ascending ASCII order: ");
for (i = 0; arr[i] != '\0'; ++i) {
printf("%c ", arr[i]);
}
printf("\n");
}
int main() {
printf("Enter a string: ");
fgets(input, sizeof(input), stdin); // 防止换行符
input[strcspn(input, "\n")] = '\0'; // 去除末尾的换行符
copy_vowels(input, output);
sort_and_output(output, strlen(output));
return 0;
}
```
阅读全文
相关推荐
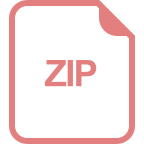















