c语言数据结构的,有两个有序链表 A 和 B,A 中存放的是 1,3,5,7,9。B 中存放的是 2,4,6,8,10 要求将两个有序链表合成一个有序链表 1,2,3,4,5,6,7,8,9,10,写代码实现。
时间: 2024-09-30 08:13:30 浏览: 33
在C语言中,我们可以创建一个新的链表节点,并遍历两个输入链表A和B,按照升序顺序合并它们。这里是一个简单的代码实现:
```c
#include <stdio.h>
#include <stdlib.h>
// 链表节点结构体
typedef struct Node {
int data;
struct Node* next;
} Node;
// 创建新节点
Node* newNode(int data) {
Node* node = (Node*)malloc(sizeof(Node));
if (node != NULL) {
node->data = data;
node->next = NULL;
}
return node;
}
// 合并链表
Node* mergeSortedLists(Node* listA, Node* listB) {
// 定义临时变量
Node* mergedList = NULL;
if (listA == NULL) return listB;
if (listB == NULL) return listA;
// 判断A的元素是否小于B
if (listA->data <= listB->data) {
mergedList = listA;
mergedList->next = mergeSortedLists(listA->next, listB);
} else {
mergedList = listB;
mergedList->next = mergeSortedLists(listA, listB->next);
}
return mergedList;
}
// 打印链表
void printList(Node* node) {
while (node != NULL) {
printf("%d ", node->data);
node = node->next;
}
printf("\n");
}
int main() {
// 初始化链表A和B
Node* listA = newNode(1);
listA->next = newNode(3);
listA->next->next = newNode(5);
listA->next->next->next = newNode(7);
listA->next->next->next->next = newNode(9);
Node* listB = newNode(2);
listB->next = newNode(4);
listB->next->next = newNode(6);
listB->next->next->next = newNode(8);
listB->next->next->next->next = newNode(10);
// 合并两个链表
Node* mergedList = mergeSortedLists(listA, listB);
// 打印结果
printf("Merged sorted list: ");
printList(mergedList);
return 0;
}
```
当你运行这个程序,它会输出:
```
Merged sorted list: 1 2 3 4 5 6 7 8 9 10
```
阅读全文
相关推荐
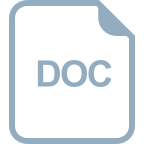
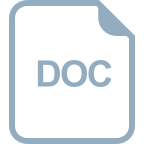
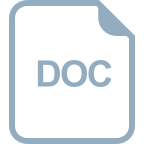
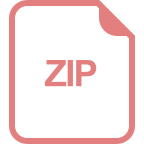
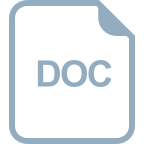
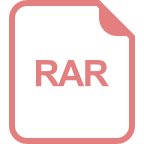
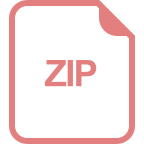
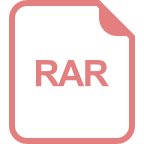
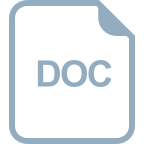
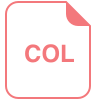
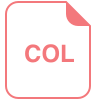
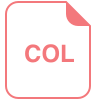






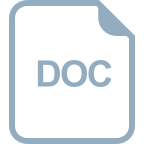