修改文件夹中图片名字,按照1,2,3等序号排列,c++代码实现
时间: 2024-10-20 09:05:05 浏览: 27
在C++中,你可以使用标准库`filesystem`来处理文件和目录操作,包括重命名文件。这里是一个简单的示例,它会遍历指定文件夹内的所有图片(假设它们都是.jpg格式),并按顺序递增数字作为新名称:
```cpp
#include <iostream>
#include <filesystem>
namespace fs = std::filesystem;
void rename_images_to_numbers(const std::string& folder_path) {
for (const auto &entry : fs::directory_iterator(folder_path)) {
if (entry.is_regular_file() && entry.path().extension() == ".jpg") {
size_t index = 0;
// 获取当前图片的原始名称,假设没有其他数字前缀
std::string original_name = entry.path().filename().stem();
// 分离原始名称中的数字部分,如果不存在则设置为0
std::smatch match;
if (std::regex_search(original_name, match, std::regex("\\d+"))) {
index = std::stoi(match[0]);
}
// 新名称添加序号,保留原名前缀
std::string new_name = original_name + "_" + std::to_string(index + 1) + ".jpg";
// 生成新的完整路径
std::string new_full_path = folder_path / new_name;
// 如果新路径还未存在,则重命名
if (!fs::exists(new_full_path)) {
fs::rename(entry.path(), new_full_path);
std::cout << "Renamed " << entry.path().filename() << " to " << new_full_path << "\n";
} else {
std::cerr << "Skip renaming because file already exists at " << new_full_path << "\n";
}
}
}
}
int main() {
try {
rename_images_to_numbers("path/to/your/folder");
} catch (const fs::filesystem_error &e) {
std::cerr << "Error: " << e.what() << '\n';
}
return 0;
}
```
在这个例子中,你需要将`"path/to/your/folder"`替换为你想要修改图片的实际文件夹路径。
阅读全文
相关推荐
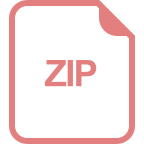

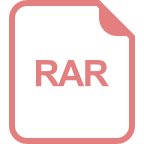
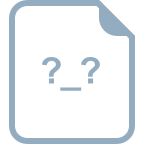
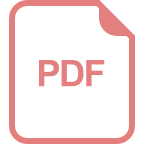
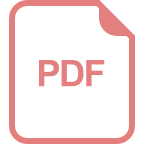
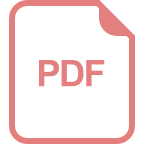
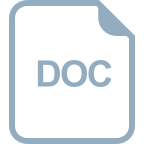
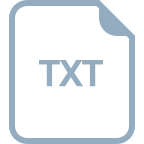
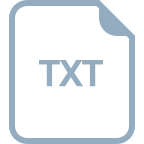
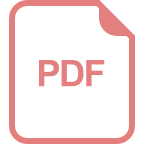
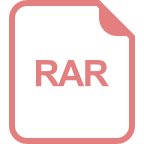
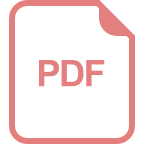

