如何用C语言实现一个简单的天神密码(Caesar Cipher)加密解密程序?
时间: 2024-10-19 12:06:15 浏览: 47
在C语言中,我们可以使用数组和循环结构来实现一个简单的凯撒密码(也称为换位加密)。凯撒密码是一种替换式加密,通过将明文中的每个字母按照特定的偏移量进行移动来加密。以下是一个基本的实现示例:
```c
#include <stdio.h>
#include <string.h>
// 加密函数
void caesar_cipher_encrypt(char *plaintext, int shift, char *ciphertext) {
int len = strlen(plaintext);
for (int i = 0; i < len; ++i) {
if (isalpha(plaintext[i])) { // 检查字符是否为字母
ciphertext[i] = ((plaintext[i] - 'A' + shift) % 26) + 'A'; // 字母表绕圈处理
} else {
ciphertext[i] = plaintext[i]; // 非字母字符不变
}
}
}
// 解密函数
void caesar_cipher_decrypt(char *ciphertext, int shift, char *plaintext) {
caesar_cipher_encrypt(ciphertext, -shift, plaintext); // 对加密后的文本进行反向加密
}
int main() {
char plaintext[100], ciphertext[100];
printf("请输入明文:");
fgets(plaintext, sizeof(plaintext), stdin);
plaintext[strlen(plaintext) - 1] = '\0'; // 去除fgets的换行符
int shift;
printf("请输入偏移量(整数):");
scanf("%d", &shift);
caesar_cipher_encrypt(plaintext, shift, ciphertext);
printf("加密后的密文:%s\n", ciphertext);
caesar_cipher_decrypt(ciphertext, shift, plaintext);
printf("解密后的原文:%s\n", plaintext);
return 0;
}
```
在这个例子中,`caesar_cipher_encrypt`用于加密,`caesar_cipher_decrypt`用于解密。用户需要输入明文、偏移量,程序会分别对输入的明文进行加密并输出密文,然后解密返回原文。
阅读全文
相关推荐
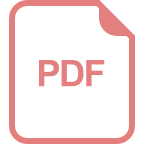
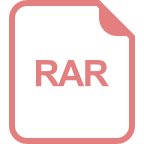
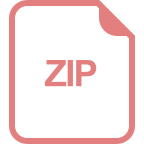

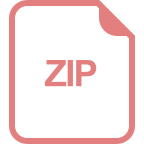
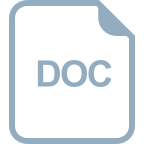
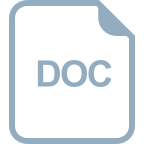
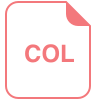





