写一个图书管理的html的代码
时间: 2024-09-18 22:11:08 浏览: 30
创建一个简单的图书管理系统HTML页面,通常包含表格用于展示书籍信息,以及输入框用于添加新书。这是一个基础示例:
```html
<!DOCTYPE html>
<html lang="zh">
<head>
<meta charset="UTF-8">
<title>图书管理系统</title>
<style>
table {
width: 100%;
border-collapse: collapse;
}
th, td {
padding: 8px;
text-align: left;
border-bottom: 1px solid #ddd;
}
tr:hover {background-color: #f5f5f5;}
input[type=text] {
margin-bottom: 10px;
}
</style>
</head>
<body>
<h1>图书列表</h1>
<table id="bookList">
<thead>
<tr>
<th>ID</th>
<th>书名</th>
<th>作者</th>
<th>操作</th>
</tr>
</thead>
<tbody>
<!-- 这里需要JavaScript填充数据 -->
</tbody>
</table>
<h2>添加新书</h2>
<form id="addBookForm">
<input type="text" id="bookId" placeholder="请输入ID">
<input type="text" id="bookName" placeholder="请输入书名">
<input type="text" id="authorName" placeholder="请输入作者名字">
<button type="submit">添加书籍</button>
</form>
<script>
// 这部分通常由服务器端数据填充或AJAX请求获取
// 这里仅作演示,实际应用需配合后台处理
let books = [
{id: 1, name: '书名1', author: '作者1'},
{id: 2, name: '书名2', author: '作者2'}
];
function displayBooks() {
books.forEach(book => {
const row = document.createElement('tr');
row.innerHTML = `
<td>${book.id}</td>
<td>${book.name}</td>
<td>${book.author}</td>
<td><a href="#">编辑</a> | <a href="#">删除</a></td>
`;
document.querySelector('#bookList tbody').appendChild(row);
});
}
document.getElementById('addBookForm').addEventListener('submit', e => {
e.preventDefault();
// 实际应用中这里会发送POST请求到服务器
console.log('添加书籍:', document.getElementById('bookId').value, document.getElementById('bookName').value, document.getElementById('authorName').value);
displayBooks(); // 渲染已有的书籍信息
});
displayBooks(); // 初始加载书籍列表
</script>
</body>
</html>
```
阅读全文
相关推荐
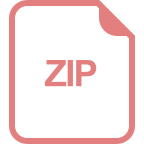
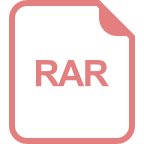
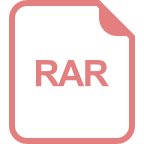
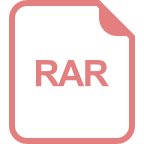
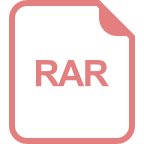
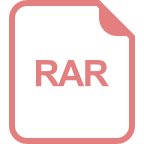
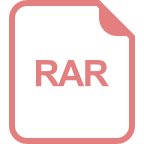
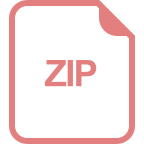
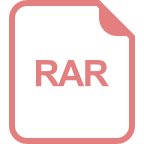
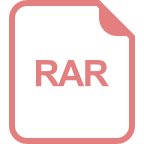
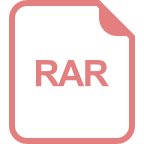
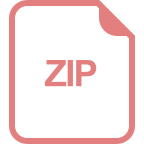
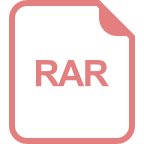
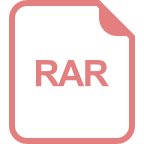
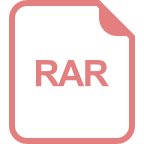
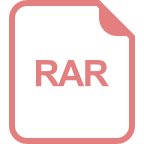
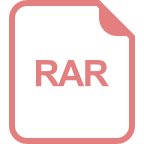
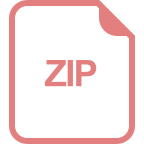
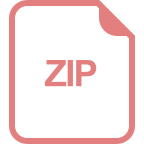