astar算法路径规划PYTHON
时间: 2024-08-14 21:10:14 浏览: 52
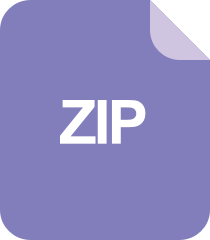
基于DWA算法和Astar算法的轮式机器人路径规划python源码+项目说明.zip
A*(A star)算法是一种启发式搜索算法,用于解决图形中的最短路径问题,特别是在寻找两个节点之间的最优路径时非常有效。它是Dijkstra算法的一种改进,结合了广度优先搜索(BFS)和贪心策略。
在Python中,你可以使用`heapq`库来实现A*算法。首先,你需要创建一个优先级队列来存储节点及其评估函数值(通常是距离目标节点的估计成本加上实际已走的距离)。然后按照这个评估函数值对节点排序,每次从队列中取出当前代价最低的节点并扩展其相邻节点。如果找到了目标节点,则返回路径;否则继续直到遍历完整个图。
以下是一个简单的A*算法的基本步骤:
1. 初始化:设置起始节点(start),目标节点(goal),以及空的集合来保存已经访问过的节点(visited set)和优先级队列(open list)。
2. 计算每个节点的f、g和h值:f值= g+heuristic(估算值),g值是从起始节点到当前节点的实际代价,h值是估算从当前节点到目标节点的最小代价。
3. 将起始节点添加到open list,并设置其g和f值。
4. 当open list不为空时,循环执行以下操作:
a. 从open list中选取f值最小的节点作为当前节点。
b. 如果当前节点是目标节点,回溯生成路径并结束。
c. 否则,将当前节点标记为已访问,更新其相邻节点的f值,然后将它们加入open list。
5. 如果未找到路径,说明没有可达的目标节点,返回无解。
阅读全文
相关推荐
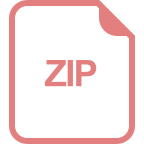
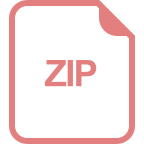
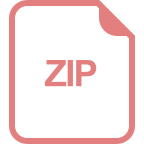
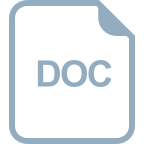

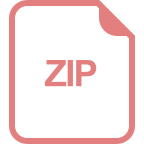
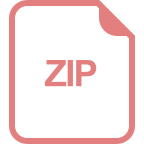
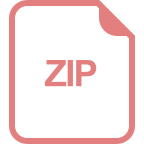
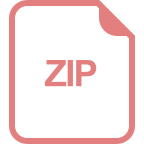
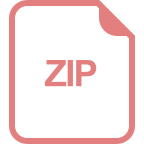
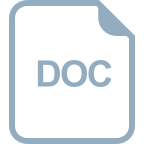
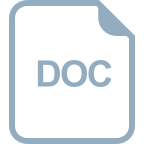
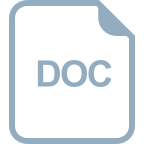
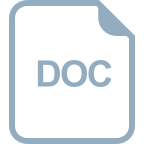


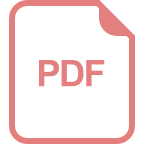
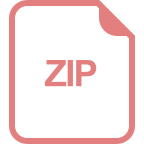