threejs 3D 地球 经纬度转换坐标
时间: 2023-08-04 09:06:07 浏览: 172
在 Three.js 中,你可以使用球体的经纬度来转换为三维坐标。下面是一个示例代码片段,展示了如何将经纬度转换为 Three.js 中的坐标:
```javascript
// 创建一个场景
const scene = new THREE.Scene();
// 创建一个相机
const camera = new THREE.PerspectiveCamera(75, window.innerWidth / window.innerHeight, 0.1, 1000);
camera.position.z = 5;
// 创建一个渲染器
const renderer = new THREE.WebGLRenderer();
renderer.setSize(window.innerWidth, window.innerHeight);
document.body.appendChild(renderer.domElement);
// 创建一个球体
const geometry = new THREE.SphereGeometry(1, 32, 32); // 半径为1,分段数为32
const material = new THREE.MeshBasicMaterial({ color: 0x00ff00 });
const earth = new THREE.Mesh(geometry, material);
scene.add(earth);
// 将经纬度转换为 Three.js 中的坐标
function latLonToVector3(lat, lon, radius) {
const phi = (lat * Math.PI) / 180;
const theta = ((lon - 180) * Math.PI) / 180;
const x = -(radius * Math.cos(phi) * Math.cos(theta));
const y = radius * Math.sin(phi);
const z = radius * Math.cos(phi) * Math.sin(theta);
return new THREE.Vector3(x, y, z);
}
// 设置地球的位置
const lat = 40; // 纬度
const lon = -100; // 经度
const radius = 5; // 球体半径
earth.position.copy(latLonToVector3(lat, lon, radius));
// 动画循环
function animate() {
requestAnimationFrame(animate);
// 使地球旋转
earth.rotation.y += 0.01;
// 渲染场景
renderer.render(scene, camera);
}
// 开始动画循环
animate();
```
在这个代码中,我们创建了一个场景、相机和渲染器,并创建了一个球体表示地球。然后,我们定义了一个 `latLonToVector3` 函数,用于将经纬度转换为 Three.js 中的坐标。通过调用这个函数并设置地球的位置,我们可以将地球放置在指定的经纬度位置上。在动画循环中,我们让地球旋转,并使用渲染器来渲染场景。
你可以根据需要自定义代码,例如改变球体的材质、调整相机位置或调整地球的半径。
阅读全文
相关推荐



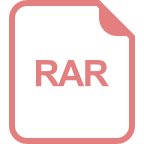











