用C语言编写一个算法函数,使用链栈或队列,判定给定的字符序列是否为回文,并验证。 提示:回文是指正读和反读均相同的字符序列。要有输入。
时间: 2024-10-15 13:27:31 浏览: 52
在C语言中,我们可以使用链栈(双向链表)或者队列来判断一个字符序列是否为回文。这里我会给出一个简单的链栈实现示例,因为链栈遍历过程更直观,可以轻松地从头开始逐个比较字符直到达到序列尾部。
```c
#include <stdio.h>
#include <stdlib.h>
// 定义节点结构体
typedef struct Node {
char data;
struct Node* next;
struct Node* prev;
} Node;
// 初始化链栈
Node* initStack() {
return (Node*)malloc(sizeof(Node));
}
// 入栈
void push(Node** top, char c) {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = c;
newNode->next = *top;
newNode->prev = NULL;
if (*top != NULL) {
(*top)->prev = newNode;
}
*top = newNode;
}
// 出栈并返回顶部元素
char pop(Node** top) {
if (*top == NULL) {
printf("Error: Stack is empty.\n");
exit(1);
}
char data = (*top)->data;
Node* temp = *top;
*top = (*top)->next;
free(temp);
return data;
}
// 检查回文
int isPalindrome(char str[]) {
Node* stackTop = initStack();
for (int i = 0; str[i] != '\0'; i++) {
push(&stackTop, str[i]);
}
// 反转字符串并与链栈顶元素对比
int j = strlen(str) - 1;
while (j >= 0 && stackTop != NULL) {
if (str[j] != pop(&stackTop)) {
return 0; // 不是回文
}
j--;
}
if (stackTop == NULL) {
return 1; // 是回文
} else {
return 0; // 栈未空,说明不是回文
}
}
int main() {
char input[100];
printf("Enter a string to check if it's a palindrome: ");
fgets(input, sizeof(input), stdin);
// 移除fgets添加的换行符
input[strlen(input) - 1] = '\0';
if (isPalindrome(input)) {
printf("%s is a palindrome.\n", input);
} else {
printf("%s is not a palindrome.\n", input);
}
return 0;
}
```
阅读全文
相关推荐
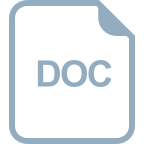
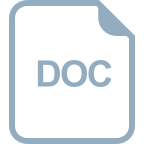
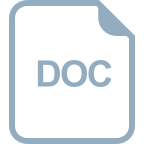















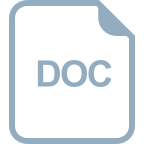