A*算法改进 python
时间: 2024-09-03 14:00:18 浏览: 53
A*算法是一种启发式搜索算法,常用于寻找两点之间最短路径的问题,特别是在图形或地图导航中。它结合了宽度优先搜索(广度优先)的优点和迪杰斯特拉算法(Dijkstra)的局部最优特性,并通过引入估价函数来加速搜索过程。
在Python中,A*算法可以使用如`heapq`库来进行优先级队列操作,因为它的效率比列表更适合处理大规模数据。下面是一个简单的A*算法的基本步骤:
1. 初始化:创建一个空的开放列表(open set)和关闭列表(closed set),以及起点(start)和终点(end)。
2. 设置起点的f值(g值+启发式值heuristic),并加入开放列表。
3. 循环直到找到终点或开放列表为空:
- 弹出当前f值最小的节点作为当前节点。
- 如果当前节点是终点,返回路径。
- 否则,将当前节点的所有邻接节点添加到开放列表中,计算它们的f、g和h值,并根据f值排序。
4. 若无解,则返回无路可达的信息。
为了改进A*算法的性能,可以考虑以下几个方面:
- **优化启发式函数**:选择一个好的估计函数,使其尽可能接近实际距离,减少搜索范围。
- **剪枝策略**:例如,当搜索空间过大时,可以设置一个启发式误差阈值,超出这个阈值的节点不再加入开放列表。
- **内存管理**:对于大型图,可以使用数据结构(如字典或集合)来存储已访问节点,而不是列表,提高查找速度。
相关问题
Theta* 算法python代码
Theta*(也称为A* with relaxation)是一种改进版的A*搜索算法,它允许在估算路径成本时使用更宽松的估价函数,这在某些情况下可以加速搜索过程。以下是使用Python实现基本的A*算法的一个简单示例,Theta*通常是通过类似的方式进行扩展:
```python
import heapq
class Node:
def __init__(self, pos, g=0, h=0, f=0):
self.pos = pos
self.g = g # 实际代价
self.h = h # 估计代价
self.f = f # 总代价 f = g + h
def __lt__(self, other):
return self.f < other.f
def theta_star(start, end, heuristic, closed_set=None, relaxed_heuristic=None):
if relaxed_heuristic is None:
relaxed_heuristic = heuristic
open_set = [Node(start)]
closed_set = [] if closed_set is None else closed_set
came_from = {}
while open_set:
current = heapq.heappop(open_set)
if current.pos == end:
path = []
while current in came_from:
path.append(current)
current = came_from[current]
path.reverse()
return path
for neighbor in get_neighbors(current.pos):
tentative_g_score = current.g + distance(current.pos, neighbor)
if neighbor not in open_set or tentative_g_score < neighbor.g:
neighbor.g = tentative_g_score
neighbor.h = relaxed_heuristic(neighbor) # 使用relaxed_heuristic
neighbor.f = neighbor.g + neighbor.h
if neighbor not in open_set:
heapq.heappush(open_set, neighbor)
came_from[neighbor] = current
return None # No path found
# 假设get_neighbors()返回给定位置的所有邻居节点
# distance()计算两点之间的实际距离
```
在这个代码中,`heuristic`是你自定义的距离启发式函数,而`relaxed_heuristic`是用于优化搜索的松弛估价函数。注意实际应用中你需要根据具体需求来实现这两个函数。
差分进化算法改进python
差分进化算法(DE)是一种优化算法,用于解决函数优化问题。它通过模拟生物进化的过程,通过变异和交叉操作来搜索最优解。在Python中,可以使用numpy库来实现差分进化算法。
以下是一个简单的差分进化算法的Python实现示例:
```python
import numpy as np
def differential_evolution(fitness_func, bounds, pop_size=50, F=0.8, CR=0.9, max_iter=100):
# 初始化种群
pop = np.random.uniform(bounds[:, 0], bounds[:, 1], (pop_size, len(bounds)))
best_solution = None
best_fitness = float('inf')
for i in range(max_iter):
for j in range(pop_size):
# 选择三个不同的个体作为变异向量
candidates = [idx for idx in range(pop_size) if idx != j]
a, b, c = np.random.choice(candidates, 3, replace=False)
# 变异操作
mutant = pop[a] + F * (pop[b] - pop[c])
# 交叉操作
trial = np.copy(pop[j])
for k in range(len(bounds)):
if np.random.rand() < CR:
trial[k] = mutant[k]
# 选择操作
trial_fitness = fitness_func(trial)
if trial_fitness < best_fitness:
best_solution = trial
best_fitness = trial_fitness
if trial_fitness <= fitness_func(pop[j]):
pop[j] = trial
return best_solution, best_fitness
# 示例适应度函数
def fitness_func(x):
return np.sum(x**2)
# 示例边界
bounds = np.array([[-5, 5], [-5, 5]])
# 运行差分进化算法
best_solution, best_fitness = differential_evolution(fitness_func, bounds)
print("Best solution:", best_solution)
print("Best fitness:", best_fitness)
```
这个示例中,我们定义了一个适应度函数`fitness_func`,它计算了解的适应度值。然后,我们定义了变量的边界`bounds`,用于限制解的取值范围。最后,我们调用`differential_evolution`函数来运行差分进化算法,并输出最佳解和最佳适应度值。
阅读全文
相关推荐
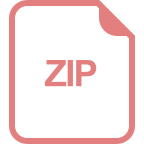
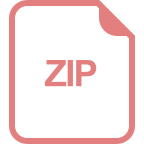
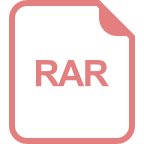
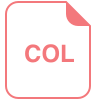
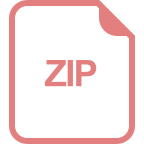
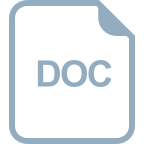
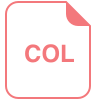
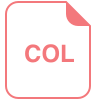
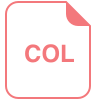
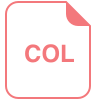
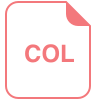
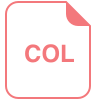
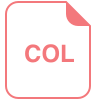
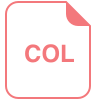
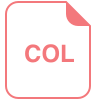
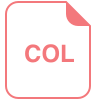
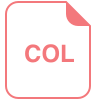
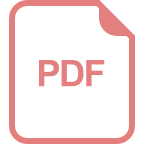