创建一个包含学生基本信息(学号、姓名、性别、专业、成绩)的线性链表
时间: 2024-10-07 13:08:45 浏览: 48
创建一个包含学生基本信息的线性链表通常涉及定义一个节点类(Node),以及一个链表类(List),这两个类的设计如下:
1. **Node** 类:
```java
class StudentNode {
int studentID; // 学号
String name; // 姓名
char gender; // 性别 ('M' or 'F')
String major; // 专业
double score; // 成绩
StudentNode(int id, String name, char gender, String major, double score) {
this.studentID = id;
this.name = name;
this.gender = gender;
this.major = major;
this.score = score;
}
// 添加指向下一个节点的引用 (link)
StudentNode next;
}
```
2. **LinkedList** 类:
```java
class StudentLinkedList {
// 链表头节点
StudentNode head;
// 插入新节点的方法
void addStudent(StudentNode newNode) {
if (head == null) {
head = newNode;
} else {
StudentNode current = head;
while (current.next != null) {
current = current.next;
}
current.next = newNode;
}
}
// 其他方法如删除节点、查找特定学生等
// ...
// 打印链表所有学生的函数
void printStudents() {
StudentNode temp = head;
while (temp != null) {
System.out.println("学号:" + temp.studentID + ",姓名:" + temp.name +
",性别:" + temp.gender + ",专业:" + temp.major + ",成绩:" + temp.score);
temp = temp.next;
}
}
}
```
阅读全文
相关推荐
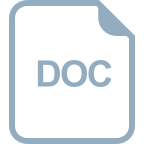
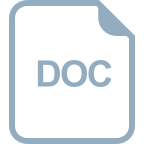
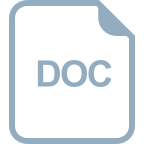


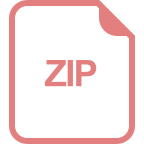
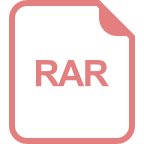
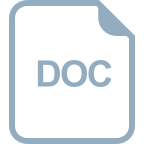
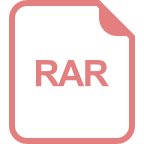
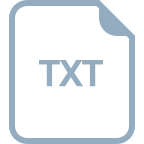
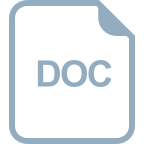
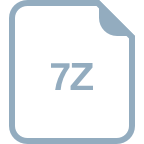
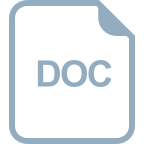
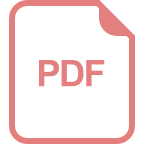
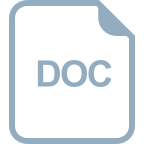
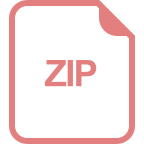
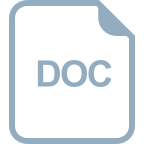
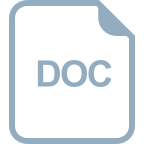
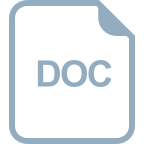