python 词云图代码示例
时间: 2023-11-14 14:12:04 浏览: 200
Python可以使用wordcloud库来实现词云图的生成。下面是一个简单的代码示例:
```
from wordcloud import WordCloud
import matplotlib.pyplot as plt
# 读取文本文件
text = open('test.txt').read()
# 生成词云图
wordcloud = WordCloud(background_color='white', width=800, height=600).generate(text)
# 显示词云图
plt.imshow(wordcloud, interpolation='bilinear')
plt.axis('off')
plt.show()
```
其中,`test.txt`是包含词语内容的文本文件,`background_color`指定词云图的背景颜色,`width`和`height`指定词云图的宽度和高度。生成的词云图可以使用`imshow`函数显示出来。
相关问题
python词云图代码
生成词云图需要使用第三方库 `wordcloud`,安装方式为在命令行输入 `pip install wordcloud`。
下面是一个简单的 Python 词云图代码示例:
```python
import jieba
from wordcloud import WordCloud
# 读取文本文件
with open('text.txt', 'r', encoding='utf-8') as f:
text = f.read()
# 使用 jieba 分词
words = jieba.cut(text)
# 拼接分词结果
cut_text = ' '.join(words)
# 生成词云图
wordcloud = WordCloud(
font_path='msyh.ttc', # 指定字体文件,可以使用本地的中文字体文件
width=800,
height=600,
background_color='white',
).generate(cut_text)
# 保存词云图
wordcloud.to_file('wordcloud.png')
```
其中,`text.txt` 是待生成词云图的文本文件,`msyh.ttc` 是中文字体文件(也可以使用其他字体文件),`wordcloud.png` 是生成的词云图保存的文件名。你可以根据实际情况修改这些参数。
python词云图代码wordcloud
下面是一个使用Python实现词云图的示例代码:
```python
import jieba
from wordcloud import WordCloud
import matplotlib.pyplot as plt
# 读取文本文件
with open('text.txt', 'r', encoding='utf-8') as f:
text = f.read()
# 使用jieba分词
words = jieba.cut(text)
# 生成词云图
wordcloud = WordCloud(font_path='msyh.ttc', background_color='white').generate(' '.join(words))
# 显示词云图
plt.imshow(wordcloud)
plt.axis('off')
plt.show()
```
这段代码中,我们首先使用`jieba`库对我们要处理的文本进行分词处理。然后使用`WordCloud`库生成词云图,使用`matplotlib`库展示我们生成的图表。其中,`font_path`参数指定了使用的字体文件路径,`background_color`参数指定了词云图的背景颜色。
阅读全文
相关推荐
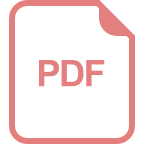











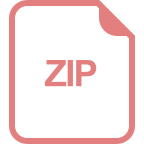
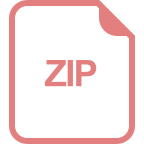