如何在Keil5集成开发环境中为STM32项目实现PID控制算法并导入至代码中?
时间: 2024-12-12 20:28:40 浏览: 17
在Keil5集成开发环境中为STM32项目实现PID (Proportional-Integral-Derivative) 控制算法通常需要以下步骤:
1. **添加头文件**:
- 包含必要的数学库,如`math.h`,以及PID控制相关的自定义头文件。
```c
#include "stm32f4xx_hal.h"
#include <stdio.h>
#include <math.h> // for math functions
```
2. **声明PID结构体**:
定义一个包含比例、积分和微分系数的结构体,以及用于存储中间计算结果的变量。
```c
typedef struct {
float kp; // Proportional gain
float ki; // Integral gain
float kd; // Derivative gain
float setpoint; // Desired output
float error; // Current error
float integral; // Cumulative integral term
float derivative; // Error derivative
float last_error; // Last error for derivative calculation
float output; // PID output
} pid_t;
```
3. **初始化PID函数**:
在程序启动时或配置阶段设置PID参数,并清零累积项。
```c
void pid_init(pid_t *pid, float kp, float ki, float kd)
{
pid->kp = kp;
pid->ki = ki;
pid->kd = kd;
pid->integral = 0.0;
pid->last_error = 0.0;
}
```
4. **PID计算函数**:
在每个想要应用PID循环的地方调用这个函数,传入当前传感器读数和设定点。
```c
void pid_update(pid_t *pid, float input, float dt)
{
float current_error = pid->setpoint - input;
pid->error = current_error;
pid->integral += current_error * dt;
if (pid->integral > PID_MAX_INTEGRAL) {
pid->integral = PID_MAX_INTEGRAL;
} else if (pid->integral < PID_MIN_INTEGRAL) {
pid->integral = PID_MIN_INTEGRAL;
}
pid->derivative = (current_error - pid->last_error) / dt;
pid->output = pid->kp * current_error + pid->ki * pid->integral + pid->kd * pid->derivative;
pid->last_error = current_error;
}
```
5. **将PID输出应用到系统**:
将PID控制器的输出转换为你系统的实际操作,比如调整电机速度或电压等。
6. **整合到STM32程序**:
在HAL初始化完成后,在主循环或其他适当的中断处理函数里调用`pid_update`。
记得在项目的`main.c`或专门的模块中调用这些函数,并考虑到实时性和资源限制,可能会需要对更新频率和计算精度进行优化。
阅读全文
相关推荐
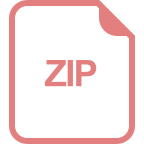
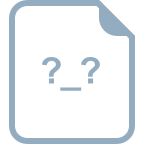
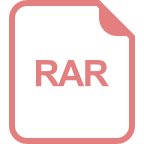
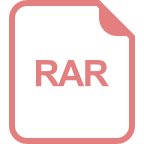
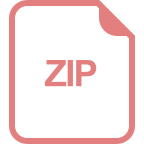
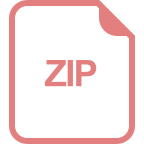
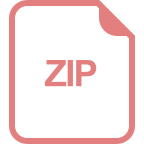
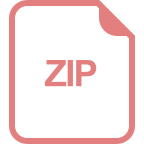
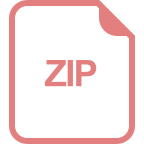
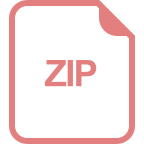
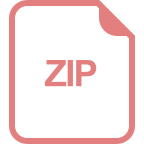
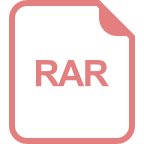
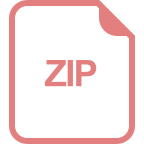
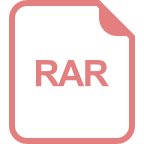
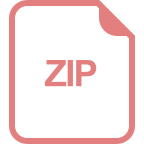
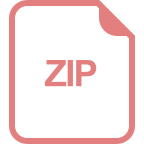
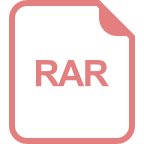