用WinAPI实现一个图片查看器:一个打开按钮,点击后打开一个打开文件对话框,打开选中的png文件显示在屏幕上,并可以用鼠标滚轮调整大小
时间: 2024-04-12 21:31:22 浏览: 149
好的,我可以为您提供一个用WinAPI实现图片查看器的示例代码。以下是一个基本的框架,您可以在此础上进行进一步的开发和改进:
```cpp
#include <Windows.h>
#include <commdlg.h>
#include <gdiplus.h>
#pragma comment(lib, "gdiplus.lib")
// 全局变量
HWND g_hWnd;
Gdiplus::Image* g_pImage = nullptr;
INT g_nImageWidth = 0;
INT g_nImageHeight = 0;
// 函数声明
LRESULT CALLBACK WndProc(HWND hWnd, UINT message, WPARAM wParam, LPARAM lParam);
BOOL OpenImageFile(HWND hWnd);
void DrawImage(HDC hdc);
void Cleanup();
// WinAPI程序入口
int WINAPI WinMain(HINSTANCE hInstance, HINSTANCE hPrevInstance, LPSTR lpCmdLine, int nCmdShow)
{
// 初始化GDI+
Gdiplus::GdiplusStartupInput gdiplusStartupInput;
ULONG_PTR gdiplusToken;
Gdiplus::GdiplusStartup(&gdiplusToken, &gdiplusStartupInput, NULL);
// 创建窗口
WNDCLASSEX wcex;
memset(&wcex, 0, sizeof(WNDCLASSEX));
wcex.cbSize = sizeof(WNDCLASSEX);
wcex.style = CS_HREDRAW | CS_VREDRAW;
wcex.lpfnWndProc = WndProc;
wcex.hInstance = hInstance;
wcex.hCursor = LoadCursor(NULL, IDC_ARROW);
wcex.hbrBackground = (HBRUSH)(COLOR_WINDOW + 1);
wcex.lpszClassName = L"ImageViewerClass";
if (!RegisterClassEx(&wcex))
return 0;
g_hWnd = CreateWindow(L"ImageViewerClass", L"图片查看器", WS_OVERLAPPEDWINDOW, CW_USEDEFAULT, CW_USEDEFAULT, 800, 600, NULL, NULL, hInstance, NULL);
if (!g_hWnd)
return 0;
ShowWindow(g_hWnd, nCmdShow);
UpdateWindow(g_hWnd);
// 消息循环
MSG msg;
while (GetMessage(&msg, NULL, 0, 0))
{
TranslateMessage(&msg);
DispatchMessage(&msg);
}
// 清理资源
Cleanup();
// 关闭GDI+
Gdiplus::GdiplusShutdown(gdiplusToken);
return (int)msg.wParam;
}
// 窗口过程
LRESULT CALLBACK WndProc(HWND hWnd, UINT message, WPARAM wParam, LPARAM lParam)
{
switch (message)
{
case WM_CREATE:
// 创建打开按钮
CreateWindow(L"BUTTON", L"打开", WS_CHILD | WS_VISIBLE | BS_PUSHBUTTON, 10, 10, 80, 30, hWnd, (HMENU)1, ((LPCREATESTRUCT)lParam)->hInstance, NULL);
break;
case WM_COMMAND:
if (LOWORD(wParam) == 1)
{
// 打开按钮被点击
OpenImageFile(hWnd);
}
break;
case WM_PAINT:
{
PAINTSTRUCT ps;
HDC hdc = BeginPaint(hWnd, &ps);
// 绘制图片
DrawImage(hdc);
EndPaint(hWnd, &ps);
}
break;
case WM_MOUSEWHEEL:
// 鼠标滚轮事件
if (g_pImage)
{
// 根据滚轮的旋转方向调整图片大小
SHORT delta = GET_WHEEL_DELTA_WPARAM(wParam);
if (delta > 0)
{
g_nImageWidth += 10;
g_nImageHeight += 10;
}
else
{
g_nImageWidth -= 10;
g_nImageHeight -= 10;
}
// 重新绘制窗口
InvalidateRect(hWnd, NULL, TRUE);
}
break;
case WM_DESTROY:
PostQuitMessage(0);
break;
default:
return DefWindowProc(hWnd, message, wParam, lParam);
}
return 0;
}
// 打开图片文件
BOOL OpenImageFile(HWND hWnd)
{
OPENFILENAME ofn;
TCHAR szFile[MAX_PATH] = { 0 };
ZeroMemory(&ofn, sizeof(ofn));
ofn.lStructSize = sizeof(ofn);
ofn.hwndOwner = hWnd;
ofn.lpstrFile = szFile;
ofn.nMaxFile = sizeof(szFile) / sizeof(*szFile);
ofn.lpstrFilter = L"PNG Files\0*.png\0All Files\0*.*\0";
ofn.nFilterIndex = 1;
ofn.Flags = OFN_PATHMUSTEXIST | OFN_FILEMUSTEXIST;
if (GetOpenFileName(&ofn))
{
// 清理旧的图片
Cleanup();
// 加载新的图片
g_pImage = Gdiplus::Image::FromFile(ofn.lpstrFile);
if (g_pImage)
{
g_nImageWidth = g_pImage->GetWidth();
g_nImageHeight = g_pImage->GetHeight();
// 重新绘制窗口
InvalidateRect(hWnd, NULL, TRUE);
}
return TRUE;
}
return FALSE;
}
// 绘制图片
void DrawImage(HDC hdc)
{
if (g_pImage)
{
Gdiplus::Graphics graphics(hdc);
// 绘制图片
graphics.DrawImage(g_pImage, 0, 0, g_nImageWidth, g_nImageHeight);
}
}
// 清理资源
void Cleanup()
{
delete g_pImage;
g_pImage = nullptr;
}
```
这个示例代码创建了一个基本的窗口,其中包含一个"打开"按钮。当点击"打开"按钮时,会打开一个文件对话框,选择一个PNG文件后,该文件将显示在窗口上。同时,您可以使用鼠标滚轮调整图片的大小。请注意,这只是一个简单的示例,可能需要进一步完善和优化。
请确保在代码中添加了必要的头文件和库文件,并将其添加到项目中以进行编译和运行。
阅读全文
相关推荐
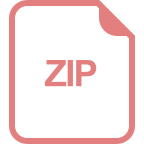
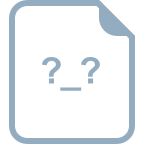


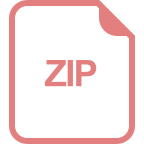
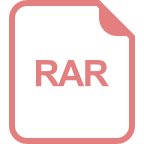
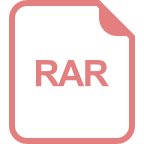
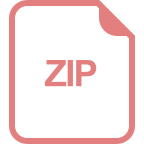
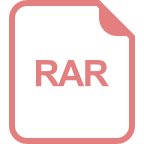
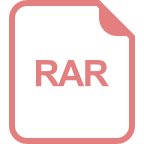
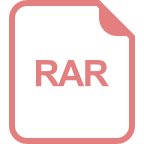
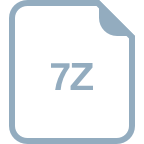
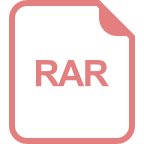
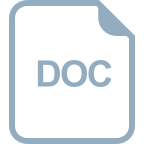
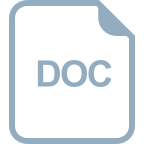