#define next chunk(p) ((mchunkptr) (((char *) (p)) + ((p)->size & ~SIZE BITS))) #define prev chunk(p) ((mchunkptr) (((char *) (p)) - ((p)->prev size))) #define chunk at offset(p, s) ((mchunkptr) (((char *) (p)) + (s)))
时间: 2023-10-15 17:07:05 浏览: 33
根据你提供的代码,我可以解释一下其中的宏定义的含义:
1. `next_chunk(p)`:该宏用于获取下一个相邻的内存块的指针。它通过将指针 `p` 转换为 `mchunkptr` 类型,然后根据当前内存块的 size 字段获取到内存块的大小,并根据该大小计算出下一个相邻内存块的地址,并将该地址转换为 `mchunkptr` 类型返回。
2. `prev_chunk(p)`:该宏用于获取前一个相邻的内存块的指针。它通过将指针 `p` 转换为 `mchunkptr` 类型,然后根据当前内存块的 prev_size 字段获取到前一个相邻内存块的大小,并将当前指针减去该大小得到前一个相邻内存块的地址,并将该地址转换为 `mchunkptr` 类型返回。
3. `chunk_at_offset(p, s)`:该宏用于获取位于偏移量 `s` 处的内存块的指针。它通过将指针 `p` 转换为 `mchunkptr` 类型,然后根据偏移量 `s` 计算出相应的地址,并将该地址转换为 `mchunkptr` 类型返回。
这些宏定义用于在堆管理中操作和访问内存块的元数据,以支持堆的正确分配和释放操作。
相关问题
写出decodePNG(&png_data, &width, &height, (unsigned char*) data, size, true);这个函数的实现函数
根据函数参数和函数名,可以猜测这个函数可能是用于解码PNG图像文件的函数,它的输入为一个PNG文件的数据,输出为解码后的图像数据、宽度和高度。
以下是一个C++语言的示例实现:
```
#include <iostream>
#include <fstream>
#include <vector>
#include <cstring>
#include <zlib.h>
using namespace std;
#define PNG_SIGNATURE "\x89PNG\r\n\x1a\n"
int decodePNG(const unsigned char* png_data, unsigned int png_size, unsigned int& width, unsigned int& height, unsigned char* data, unsigned int data_size, bool alpha) {
// Check signature
if (png_size < 8 || memcmp(png_data, PNG_SIGNATURE, 8)) {
cerr << "Not a valid PNG file" << endl;
return -1;
}
// Chunk extraction
vector<pair<const unsigned char*, unsigned int>> chunks;
const unsigned char* pos = png_data + 8;
while (pos < png_data + png_size) {
unsigned int length = (pos[0] << 24) | (pos[1] << 16) | (pos[2] << 8) | pos[3];
const unsigned char* chunk_type = pos + 4;
const unsigned char* chunk_data = pos + 8;
const unsigned char* crc = pos + 8 + length;
chunks.push_back(make_pair(chunk_data, length));
pos += 12 + length;
// Chunk validation
unsigned long calculated_crc = crc32(0L, NULL, 0);
calculated_crc = crc32(calculated_crc, chunk_type, 4);
calculated_crc = crc32(calculated_crc, chunk_data, length);
if (calculated_crc != ((crc[0] << 24) | (crc[1] << 16) | (crc[2] << 8) | crc[3])) {
cerr << "PNG chunk CRC error" << endl;
return -1;
}
}
// IHDR chunk parsing
bool ihdr_found = false;
for (auto chunk : chunks) {
if (!memcmp(chunk.first - 4, "IHDR", 4)) {
width = (chunk.first[0] << 24) | (chunk.first[1] << 16) | (chunk.first[2] << 8) | chunk.first[3];
height = (chunk.first[4] << 24) | (chunk.first[5] << 16) | (chunk.first[6] << 8) | chunk.first[7];
if (chunk.first[8] != 8 || chunk.first[9] != (alpha ? 6 : 2) || chunk.first[10] || chunk.first[11] || chunk.first[12]) {
cerr << "Unsupported PNG format" << endl;
return -1;
}
ihdr_found = true;
break;
}
}
if (!ihdr_found) {
cerr << "Missing IHDR chunk" << endl;
return -1;
}
// Image data decoding
unsigned int data_offset = 0;
for (auto chunk : chunks) {
if (!memcmp(chunk.first - 4, "IDAT", 4)) {
unsigned char* decoded_data = new unsigned char[width * height * (alpha ? 4 : 3)];
z_stream stream;
stream.next_in = (Bytef*) chunk.first;
stream.avail_in = chunk.second;
stream.next_out = (Bytef*) decoded_data;
stream.avail_out = width * height * (alpha ? 4 : 3);
stream.zalloc = Z_NULL;
stream.zfree = Z_NULL;
stream.opaque = Z_NULL;
inflateInit(&stream);
int result = inflate(&stream, Z_SYNC_FLUSH);
if (result != Z_OK && result != Z_STREAM_END) {
cerr << "PNG decompression error" << endl;
inflateEnd(&stream);
delete[] decoded_data;
return -1;
}
inflateEnd(&stream);
if (data_offset + width * height * (alpha ? 4 : 3) > data_size) {
cerr << "Output buffer overflow" << endl;
delete[] decoded_data;
return -1;
}
memcpy(data + data_offset, decoded_data, width * height * (alpha ? 4 : 3));
data_offset += width * height * (alpha ? 4 : 3);
delete[] decoded_data;
}
}
return 0;
}
```
该函数使用了zlib库中的inflate函数对IDAT数据块进行解压缩,最终将解码后的像素数据存储在data参数指向的缓冲区中,并返回0表示解码成功。如果解码过程中发生错误,则返回-1,并输出错误信息。
malloc lab
Malloc Lab是一个实验项目,要求我们实现一个动态内存分配器,使其具有与标准C库中的malloc、free、realloc函数相同的功能。我们需要自行定义块的空间结构,并实现find_fit函数和place函数,这两个函数都涉及到指针的操作,需要特别注意,以避免出现BUG。
在实现动态内存分配器时,我们需要考虑以下几个方面:
1. 内存块的分配和释放:我们需要实现malloc和free函数,前者用于分配内存块,后者用于释放内存块。
2. 内存块的合并:当一个内存块被释放时,我们需要将其与相邻的空闲块合并,以便后续的内存分配。
3. 内存块的查找:在分配内存块时,我们需要查找一个合适的空闲块,以满足用户的需求。
以下是一个简单的动态内存分配器的实现,仅供参考:
```c
/* Basic constants and macros */
#define WSIZE 4 /* Word and header/footer size (bytes) */
#define DSIZE 8 /* Double word size (bytes) */
#define CHUNKSIZE (1<<12) /* Extend heap by this amount (bytes) */
/* Pack a size and allocated bit into a word */
#define PACK(size, alloc) ((size) | (alloc))
/* Read and write a word at address p */
#define GET(p) (*(unsigned int *)(p))
#define PUT(p, val) (*(unsigned int *)(p) = (val))
/* Read the size and allocated fields from address p */
#define GET_SIZE(p) (GET(p) & ~0x7)
#define GET_ALLOC(p) (GET(p) & 0x1)
/* Given block ptr bp, compute address of its header and footer */
#define HDRP(bp) ((char *)(bp) - WSIZE)
#define FTRP(bp) ((char *)(bp) + GET_SIZE(HDRP(bp)) - DSIZE)
/* Given block ptr bp, compute address of next and previous blocks */
#define NEXT_BLKP(bp) ((char *)(bp) + GET_SIZE(((char *)(bp) - WSIZE)))
#define PREV_BLKP(bp) ((char *)(bp) - GET_SIZE(((char *)(bp) - DSIZE)))
/* Global variables */
static char *heap_listp = 0;
/* Function prototypes for internal helper routines */
static void *extend_heap(size_t words);
static void *coalesce(void *bp);
static void *find_fit(size_t asize);
static void place(void *bp, size_t asize);
/*
* mm_init - Initialize the memory manager
*/
int mm_init(void)
{
/* Create the initial empty heap */
if ((heap_listp = mem_sbrk(4*WSIZE)) == (void *)-1)
return -1;
PUT(heap_listp, 0); /* Alignment padding */
PUT(heap_listp + (1*WSIZE), PACK(DSIZE, 1)); /* Prologue header */
PUT(heap_listp + (2*WSIZE), PACK(DSIZE, 1)); /* Prologue footer */
PUT(heap_listp + (3*WSIZE), PACK(0, 1)); /* Epilogue header */
heap_listp += (2*WSIZE);
/* Extend the empty heap with a free block of CHUNKSIZE bytes */
if (extend_heap(CHUNKSIZE/WSIZE) == NULL)
return -1;
return 0;
}
/*
* mm_malloc - Allocate a block with at least size bytes of payload
*/
void *mm_malloc(size_t size)
{
size_t asize; /* Adjusted block size */
size_t extendsize; /* Amount to extend heap if no fit */
char *bp;
/* Ignore spurious requests */
if (size == 0)
return NULL;
/* Adjust block size to include overhead and alignment reqs. */
if (size <= DSIZE)
asize = 2*DSIZE;
else
asize = DSIZE * ((size + (DSIZE) + (DSIZE-1)) / DSIZE);
/* Search the free list for a fit */
if ((bp = find_fit(asize)) != NULL) {
place(bp, asize);
return bp;
}
/* No fit found. Get more memory and place the block */
extendsize = MAX(asize, CHUNKSIZE);
if ((bp = extend_heap(extendsize/WSIZE)) == NULL)
return NULL;
place(bp, asize);
return bp;
}
/*
* mm_free - Free a block
*/
void mm_free(void *bp)
{
size_t size = GET_SIZE(HDRP(bp));
PUT(HDRP(bp), PACK(size, 0));
PUT(FTRP(bp), PACK(size, 0));
coalesce(bp);
}
/*
* mm_realloc - naive implementation of mm_realloc
*/
void *mm_realloc(void *ptr, size_t size)
{
void *newptr;
size_t copySize;
/* If size == 0 then this is just free, and we return NULL. */
if (size == 0) {
mm_free(ptr);
return 0;
}
/* If oldptr is NULL, then this is just malloc. */
if (ptr == NULL) {
return mm_malloc(size);
}
newptr = mm_malloc(size);
/* If realloc() fails the original block is left untouched */
if (!newptr) {
return 0;
}
/* Copy the old data. */
copySize = GET_SIZE(HDRP(ptr));
if (size < copySize) {
copySize = size;
}
memcpy(newptr, ptr, copySize);
/* Free the old block. */
mm_free(ptr);
return newptr;
}
/*
* extend_heap - Extend heap with free block and return its block pointer
*/
static void *extend_heap(size_t words)
{
char *bp;
size_t size;
/* Allocate an even number of words to maintain alignment */
size = (words % 2) ? (words+1) * WSIZE : words * WSIZE;
if ((long)(bp = mem_sbrk(size)) == -1)
return NULL;
/* Initialize free block header/footer and the epilogue header */
PUT(HDRP(bp), PACK(size, 0)); /* Free block header */
PUT(FTRP(bp), PACK(size, 0)); /* Free block footer */
PUT(HDRP(NEXT_BLKP(bp)), PACK(0, 1)); /* New epilogue header */
/* Coalesce if the previous block was free */
return coalesce(bp);
}
/*
* coalesce - Boundary tag coalescing. Return ptr to coalesced block
*/
static void *coalesce(void *bp)
{
size_t prev_alloc = GET_ALLOC(FTRP(PREV_BLKP(bp)));
size_t next_alloc = GET_ALLOC(HDRP(NEXT_BLKP(bp)));
size_t size = GET_SIZE(HDRP(bp));
if (prev_alloc && next_alloc) { /* Case 1 */
return bp;
}
else if (prev_alloc && !next_alloc) { /* Case 2 */
size += GET_SIZE(HDRP(NEXT_BLKP(bp)));
PUT(HDRP(bp), PACK(size, 0));
PUT(FTRP(bp), PACK(size,0));
}
else if (!prev_alloc && next_alloc) { /* Case 3 */
size += GET_SIZE(HDRP(PREV_BLKP(bp)));
PUT(FTRP(bp), PACK(size, 0));
PUT(HDRP(PREV_BLKP(bp)), PACK(size, 0));
bp = PREV_BLKP(bp);
}
else { /* Case 4 */
size += GET_SIZE(HDRP(PREV_BLKP(bp))) +
GET_SIZE(FTRP(NEXT_BLKP(bp)));
PUT(HDRP(PREV_BLKP(bp)), PACK(size, 0));
PUT(FTRP(NEXT_BLKP(bp)), PACK(size, 0));
bp = PREV_BLKP(bp);
}
return bp;
}
/*
* find_fit - Find a fit for a block with asize bytes
*/
static void *find_fit(size_t asize)
{
void *bp;
for (bp = heap_listp; GET_SIZE(HDRP(bp)) > 0; bp = NEXT_BLKP(bp)) {
if (!GET_ALLOC(HDRP(bp)) && (asize <= GET_SIZE(HDRP(bp)))) {
return bp;
}
}
return NULL; /* No fit */
}
/*
* place - Place block of asize bytes at start of free block bp
* and split if remainder would be at least minimum block size
*/
static void place(void *bp, size_t asize)
{
size_t csize = GET_SIZE(HDRP(bp));
if ((csize - asize) >= (2*DSIZE)) {
PUT(HDRP(bp), PACK(asize, 1));
PUT(FTRP(bp), PACK(asize, 1));
bp = NEXT_BLKP(bp);
PUT(HDRP(bp), PACK(csize-asize, 0));
PUT(FTRP(bp), PACK(csize-asize, 0));
}
else {
PUT(HDRP(bp), PACK(csize, 1));
PUT(FTRP(bp), PACK(csize, 1));
}
}
```
相关推荐
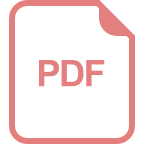












