如何用Python编写并实现具有粒子效果的爱心形状代码?
时间: 2024-12-13 09:27:21 浏览: 50
在Python中,我们可以使用pygame库来模拟粒子效果并生成爱心形状。下面是一个简单的示例,它使用了random模块来随机移动和改变颜色的粒子。这个例子并不是非常复杂,但展示了基本的概念。
```python
import pygame
import random
# 初始化Pygame
pygame.init()
# 设置窗口大小和背景色
screen = pygame.display.set_mode((600, 600))
background_color = (255, 255, 255)
pygame.display.set_caption("爱心粒子")
# 定义一个粒子类
class Particle:
def __init__(self, x, y, size, color):
self.x = x
self.y = y
self.size = size
self.color = color
self.speed = [random.uniform(-2, 2), random.uniform(-2, 2)]
def update(self):
self.x += self.speed[0]
self.y += self.speed[1]
def render(self):
pygame.draw.circle(screen, self.color, (int(self.x), int(self.y)), self.size)
# 创建爱心形状
def draw_heart(particles):
for particle in particles:
if particle.x > screen.get_width() or particle.x < 0:
particle.speed[0] = -particle.speed[0]
if particle.y > screen.get_height() or particle.y < 0:
particle.speed[1] = -particle.speed[1]
particle.render()
# 主游戏循环
particles = [Particle(50, 50, 5, (255, 0, 0)) for _ in range(100)]
while True:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
quit()
# 更新粒子位置
for particle in particles:
particle.update()
# 清除屏幕
screen.fill(background_color)
# 绘制爱心和粒子
draw_heart(particles)
# 更新屏幕
pygame.display.flip()
```
这个代码将生成许多红色小点,并让它们沿着随机方向移动,模拟出爱心的粒子效果。
阅读全文
相关推荐
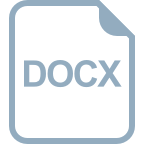
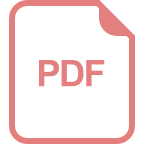
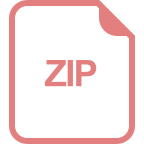















