径向基神经网络模型代码
时间: 2024-04-14 10:22:59 浏览: 14
径向基神经网络(Radial Basis Function Neural Network,RBFNN)是一种基于径向基函数的神经网络模型。下面是一个简单的径向基神经网络模型的代码示例:
```python
import numpy as np
class RBFNN:
def __init__(self, num_centers, learning_rate=0.1):
self.num_centers = num_centers
self.learning_rate = learning_rate
self.centers = None
self.weights = None
def fit(self, X, y):
# 随机选择中心点
self.centers = X[np.random.choice(X.shape[0], self.num_centers, replace=False)]
# 计算径向基函数的宽度
widths = np.linalg.norm(X[:, np.newaxis] - self.centers, axis=2)
sigma = np.mean(widths)
# 计算设计矩阵
design_matrix = np.exp(-0.5 * (widths / sigma) ** 2)
# 添加偏置项
design_matrix = np.hstack((design_matrix, np.ones((X.shape[0], 1))))
# 计算权重
self.weights = np.linalg.pinv(design_matrix) @ y
def predict(self, X):
widths = np.linalg.norm(X[:, np.newaxis] - self.centers, axis=2)
sigma = np.mean(widths)
design_matrix = np.exp(-0.5 * (widths / sigma) ** 2)
design_matrix = np.hstack((design_matrix, np.ones((X.shape[0], 1))))
y_pred = design_matrix @ self.weights
return y_pred
# 示例用法
X_train = np.array([[0], [1], [2], [3], [4], [5]])
y_train = np.array([0, 0, 1, 1, 0, 0])
rbfnn = RBFNN(num_centers=2)
rbfnn.fit(X_train, y_train)
X_test = np.array([[1.5], [3.5]])
y_pred = rbfnn.predict(X_test)
print(y_pred)
```
这段代码实现了一个简单的径向基神经网络模型。首先,在`fit`方法中,通过随机选择中心点,并计算径向基函数的宽度,构建设计矩阵,并使用伪逆求解权重。然后,在`predict`方法中,根据输入数据计算径向基函数的值,并使用权重进行预测。最后,通过示例用法展示了如何使用该模型进行训练和预测。
相关推荐
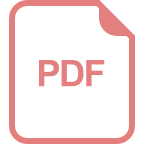














