unordered_map c实现
时间: 2023-10-17 18:37:36 浏览: 146
unordered_map 是 C++ 标准库中的一个容器,用于存储键值对,并支持快速的插入、删除和查找操作。C++ 的 unordered_map 可以通过哈希表来实现。
要在 C 中实现一个类似 unordered_map 的数据结构,你可以使用哈希表和链表的组合。下面是一个简单的示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define TABLE_SIZE 100
// 哈希表中的节点
typedef struct Node {
char key[20];
int value;
struct Node* next;
} Node;
// 哈希表
typedef struct HashMap {
Node* table[TABLE_SIZE];
} HashMap;
// 计算哈希值
int hash(const char* key) {
int hash = 0;
while (*key) {
hash += *key;
key++;
}
return hash % TABLE_SIZE;
}
// 初始化哈希表
HashMap* HashMap_Create() {
HashMap* map = (HashMap*)malloc(sizeof(HashMap));
memset(map->table, 0, sizeof(map->table));
return map;
}
// 向哈希表中插入键值对
void HashMap_Insert(HashMap* map, const char* key, int value) {
int index = hash(key);
Node* newNode = (Node*)malloc(sizeof(Node));
strcpy(newNode->key, key);
newNode->value = value;
newNode->next = NULL;
if (map->table[index] == NULL) {
map->table[index] = newNode;
} else {
Node* current = map->table[index];
while (current->next != NULL) {
current = current->next;
}
current->next = newNode;
}
}
// 从哈希表中删除指定键的节点
void HashMap_Remove(HashMap* map, const char* key) {
int index = hash(key);
Node* current = map->table[index];
Node* previous = NULL;
while (current != NULL && strcmp(current->key, key) != 0) {
previous = current;
current = current->next;
}
if (current == NULL) {
return; // 未找到指定键的节点
}
if (previous == NULL) {
map->table[index] = current->next;
} else {
previous->next = current->next;
}
free(current);
}
// 从哈希表中获取指定键对应的值
int HashMap_Get(HashMap* map, const char* key) {
int index = hash(key);
Node* current = map->table[index];
while (current != NULL && strcmp(current->key, key) != 0) {
current = current->next;
}
if (current == NULL) {
return -1; // 未找到指定键的节点
}
return current->value;
}
// 销毁哈希表
void HashMap_Destroy(HashMap* map) {
for (int i = 0; i < TABLE_SIZE; i++) {
Node* current = map->table[i];
while (current != NULL) {
Node* next = current->next;
free(current);
current = next;
}
}
free(map);
}
int main() {
HashMap* map = HashMap_Create();
HashMap_Insert(map, "key1", 1);
HashMap_Insert(map, "key2", 2);
HashMap_Insert(map, "key3", 3);
printf("value for key1: %d\n", HashMap_Get(map, "key1"));
printf("value for key2: %d\n", HashMap_Get(map, "key2"));
printf("value for key3: %d\n", HashMap_Get(map, "key3"));
HashMap_Remove(map, "key2");
printf("value for key2: %d\n", HashMap_Get(map, "key2"));
HashMap_Destroy(map);
return 0;
}
```
这个示例演示了如何使用哈希表和链表组合来实现一个类似 unordered_map 的数据结构。你可以根据实际需求进行修改和扩展。
阅读全文
相关推荐
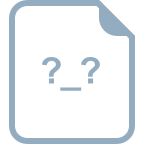
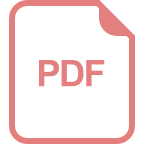
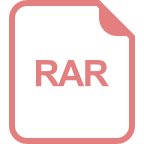















