c加加unordered_map用法
时间: 2023-10-12 18:21:35 浏览: 58
unordered_map是C++11中新增的一种关联容器,底层实现是哈希表,可以用于存储键值对。使用unordered_map需要包含头文件<unordered_map>。
下面是unordered_map的基本用法:
1. 创建unordered_map对象
```c++
std::unordered_map<std::string, int> map; //创建一个空的unordered_map对象
```
2. 插入元素
```c++
map.insert({"apple", 2}); //使用insert()函数插入元素
map["banana"] = 3; //使用下标运算符插入元素
```
3. 删除元素
```c++
map.erase("apple"); //使用erase()函数删除元素
```
4. 查找元素
```c++
auto it = map.find("banana"); //使用find()函数查找元素
if (it != map.end()) {
std::cout << "The value of banana is " << it->second << std::endl;
}
```
5. 遍历unordered_map
```c++
for (auto it = map.begin(); it != map.end(); ++it) {
std::cout << it->first << ": " << it->second << std::endl;
}
```
6. 访问元素
```c++
int value = map["banana"]; //使用下标运算符访问元素
```
需要注意的是,unordered_map中的元素是无序的,并且不允许键重复。如果需要保持键的顺序,可以使用std::map。
相关推荐
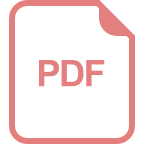
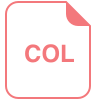
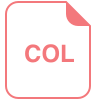
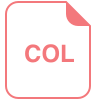
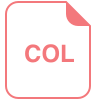
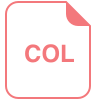









