c++生态分布生成随机数
时间: 2023-11-30 18:41:38 浏览: 91
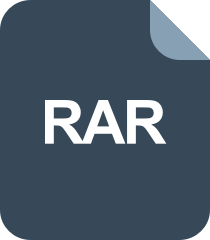
基于C++Python的开源框架.rar
为了在C++中生成正态分布的随机数,可以使用C++标准库中的`<random>`头文件中的`normal_distribution`类。该类的构造函数需要两个参数:均值和标准差。然后,可以使用该类的`operator()`方法生成正态分布的随机数。
以下是一个示例代码:
```c++
#include <iostream>
#include <random>
int main() {
// 设置随机数生成器
std::random_device rd;
std::mt19937 gen(rd());
// 设置正态分布的均值和标准差
double mean = 0.0;
double stddev = 1.0;
std::normal_distribution<double> dist(mean, stddev);
// 生成正态分布的随机数
for (int i = 0; i < 10; ++i) {
std::cout << dist(gen) << std::endl;
}
return 0;
}
```
该代码使用`std::random_device`生成随机数种子,然后使用`std::mt19937`作为随机数生成器。接下来,设置正态分布的均值和标准差,并使用`std::normal_distribution`类生成正态分布的随机数。最后,使用`operator()`方法生成随机数并输出。
阅读全文
相关推荐
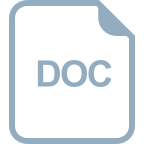
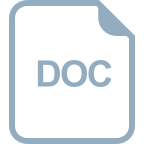
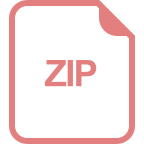
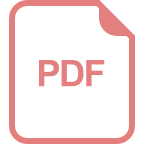
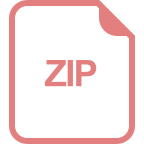
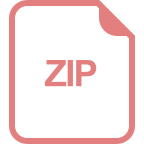
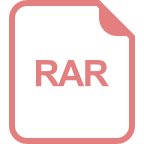
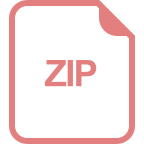
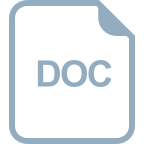
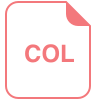
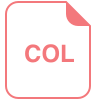
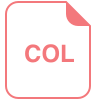
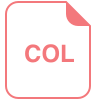
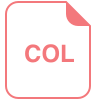


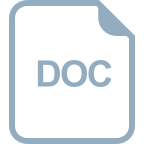
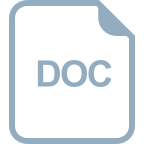