帮我用python写一个根据边缘划分前景和背景的程序
时间: 2023-10-21 07:03:46 浏览: 86
当涉及到根据图像边缘划分前景和背景时,可以使用基于边缘检测的方法,例如Canny边缘检测算法。下面是一个使用Python和OpenCV库实现的示例程序:
```python
import cv2
import numpy as np
def segment_image(image_path):
# 读取图像
image = cv2.imread(image_path)
# 转换为灰度图像
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# 使用Canny边缘检测算法检测边缘
edges = cv2.Canny(gray, 100, 200)
# 对边缘进行膨胀操作,以填充边缘间的空隙
kernel = np.ones((5, 5), np.uint8)
dilation = cv2.dilate(edges, kernel, iterations=1)
# 使用腐蚀操作,去除边缘外的噪音点
erosion = cv2.erode(dilation, kernel, iterations=1)
# 使用边缘图像作为掩码,将原始图像分割为前景和背景
segmented_image = np.zeros_like(image)
segmented_image[erosion != 0] = image[erosion != 0]
# 显示分割结果
cv2.imshow("Segmented Image", segmented_image)
cv2.waitKey(0)
cv2.destroyAllWindows()
# 示例用法
image_path = "path_to_your_image.jpg"
segment_image(image_path)
```
请确保在运行代码之前安装了OpenCV库。你需要将`image_path`变量设置为你要处理的图像的路径。该程序将加载图像,执行边缘检测并使用边缘图像作为掩码来分割前景和背景,最后显示分割结果。
阅读全文
相关推荐
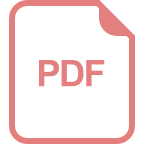
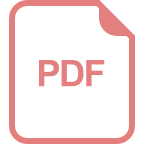
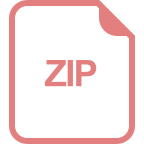
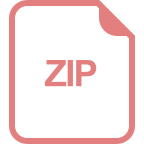
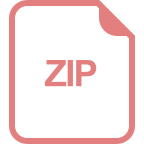
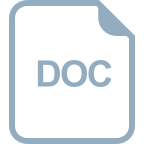
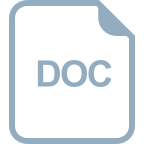
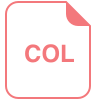
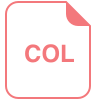
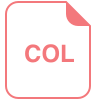
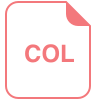
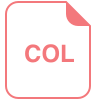
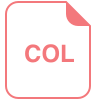
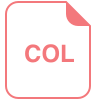
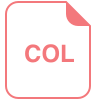
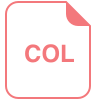
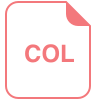
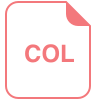